I believe everyone should build. Building is the only real validation of our ideas. There is no better way to learn.
Today, that usually means software. I think of software as today's woodworking. Today's metalsmithing.
Software is about expression. If you want to express your ideas with more than just form, you need to add function.
Let's get started.
There are a few high-level pieces that go into building all applications:
- a page
- styles
- layouts
- interactive elements
- events
- server
- fetching/storing data
Let's cover these:
We will use JavaScript and Python libraries.
I will choose Azle for front-end stuff and Flask for back-end stuff.
Let's start with our page. Pages always have an index.html file
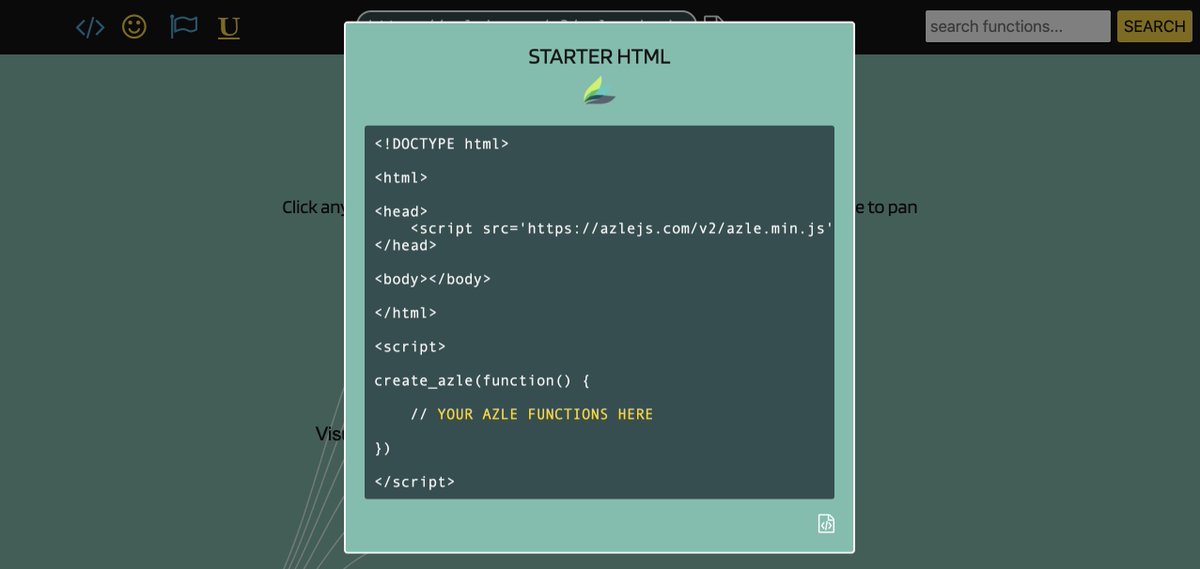
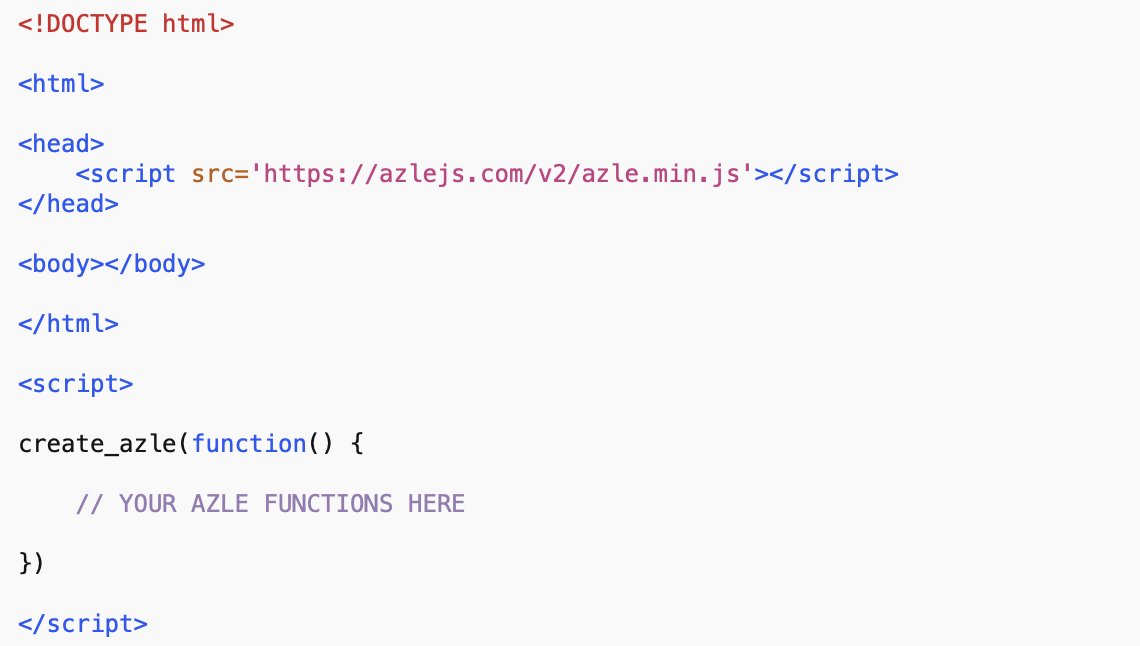
Nothing says I'm modern like Russian pastel:
flatuicolors.com/palette/ru
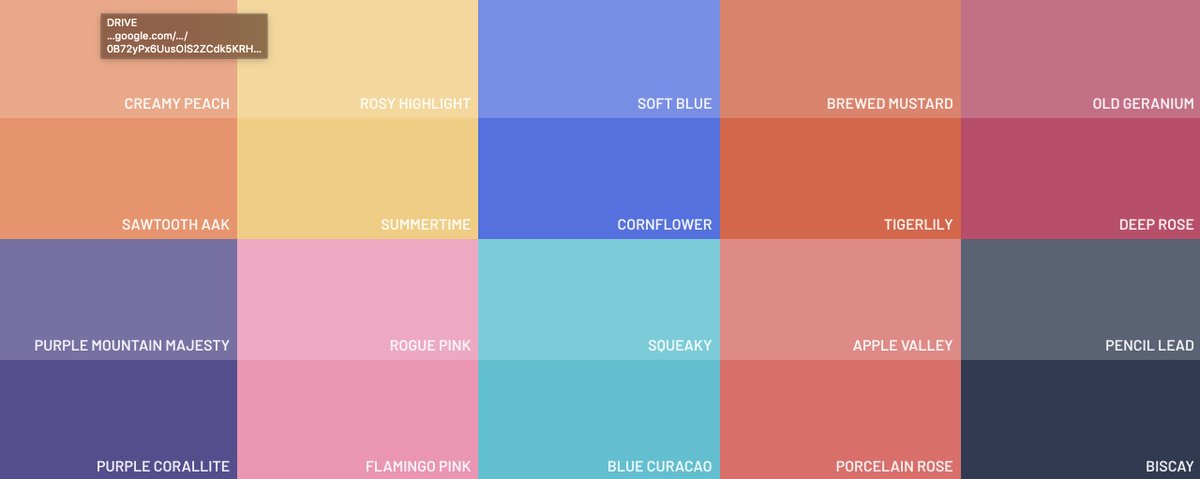
I'll choose Biscay.
We also don't want to use standard browser fonts because the only thing worse than looking like Reddit is looking like Craigslist.
fonts.google.com
I'll choose Ubuntu. Nice and modern looking.
Our style_body function now looks like this:
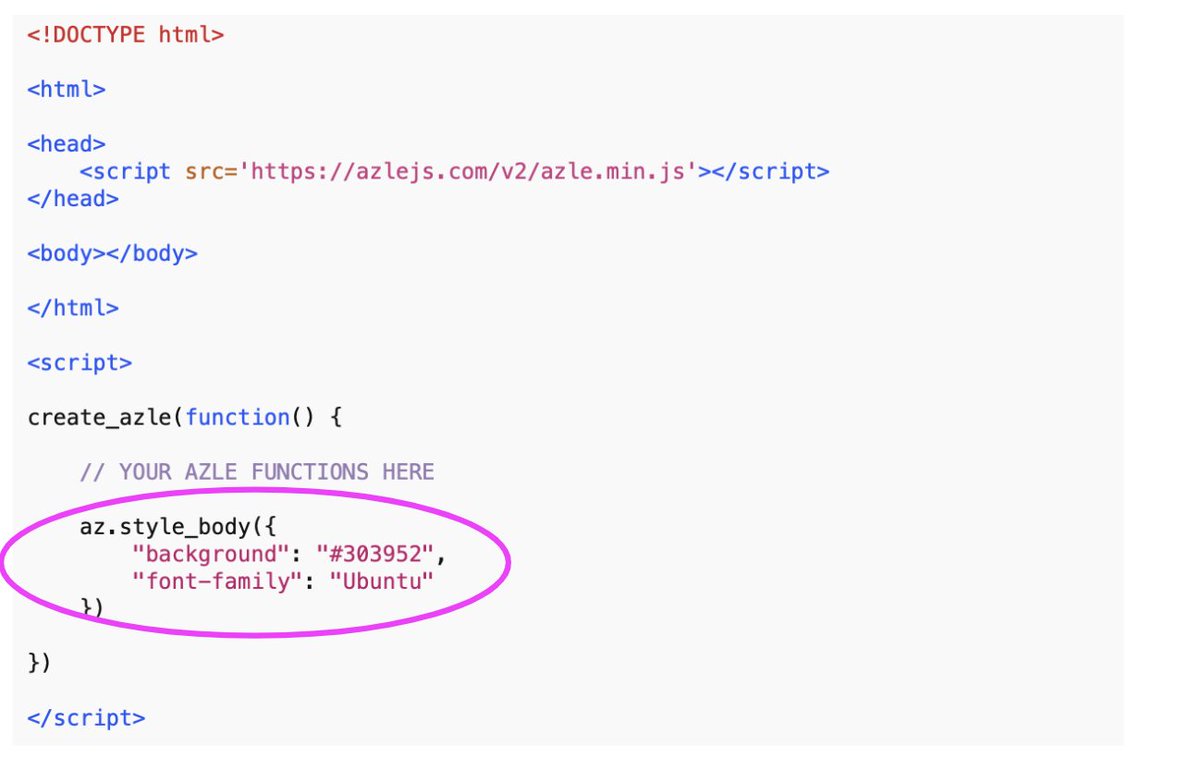
We didn't start with any mockups of what we wanted to create, which is fine. But now might be a good time to think about what we want this to be.
While I write this, covid-19 is on everyone's mind. Let's fetch covid-19 data and use a model to predict cases.
Don't be an idiot and use this model to make real-life decisions regarding a pandemic. This is just for demonstration purposes.
REST APIs deliver data and functionality "over the wire" into our browser, making it easier to create interesting applications without having to write a ton of code.
I found one here:
about-corona.net
It's free and requires no authentication. Just what we want for playing around.
After reviewing the documentation I found the endpoint we will use:
corona-api.com/timeline
This gives global counts for deaths, confirmed and recovered cases.
corona-api.com/timeline
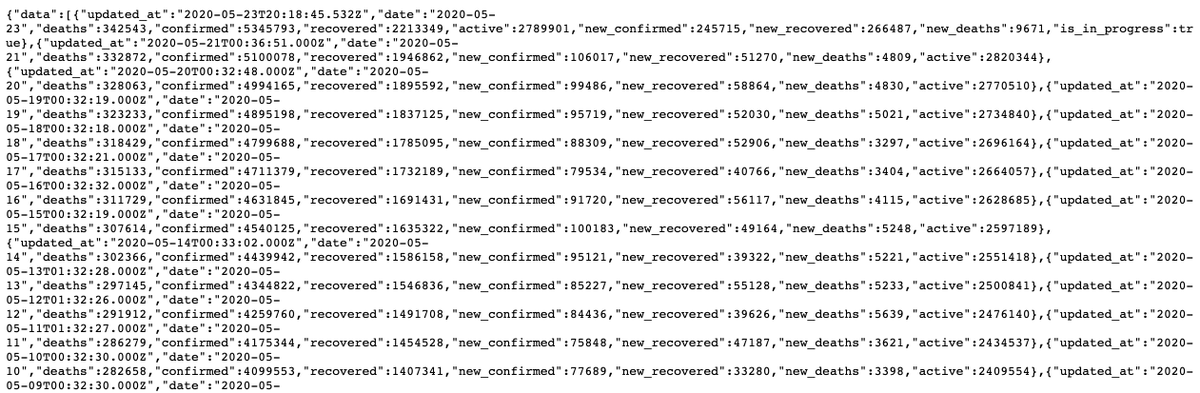
But first, let's get back to our mockup. Now that we've seen the data, we can think about how our app might look and act.
Now let's add our UI elements inside these new cells. From Azle's documentation we can grab the code we need.
For the line chart we will use another library called Plotly.
plotly.com/javascript/
Plotly is built on top of D3.js, an industry-standard visualization library written in Javascript.
Click the line chart option on Plotly's website:
Grab the line chart code and paste it into our app:
An important fact about building apps that rely on fetched data is the data must be available prior to using it.
As an example, when our users first load our app we want to show the line chart. But that line chart depends on data being available.
Asynchronous code WAITS until something has happened (e.g. data has been fetched) before calling a function of our choice.
JavaScript makes all this possible via its "fetch" API. With fetch, we simply point to the URL provided by the REST service and tell it what to do once data is received.
How do we use it? I found this:
mzl.la/2ysL4pm
...which shows us how:
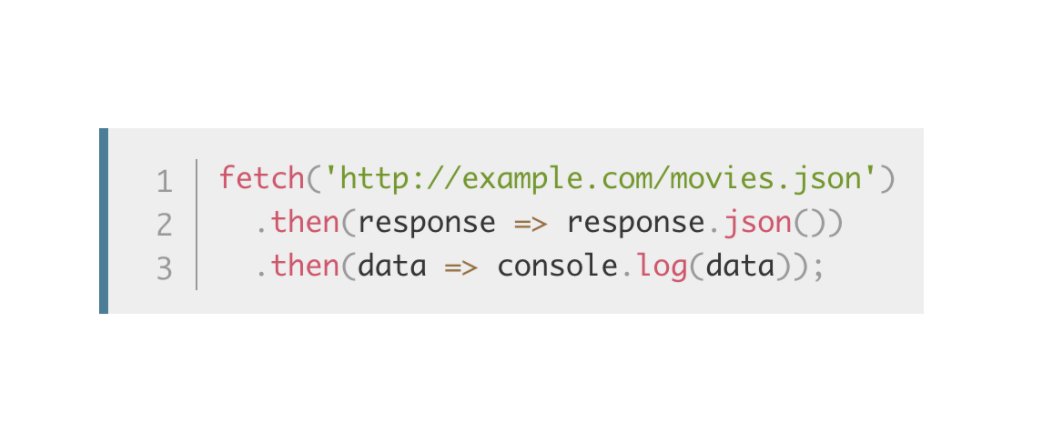
Open the browser console by right-clicking anywhere on the screen and clicking "inspect".
Then click console.
Click repeatedly into this object to view its structure:
Now we can parse this data object, using its contents to populate our line chart.
To do this we'll need to make a slight change to how our line chart is being drawn.
We need to:
1. understand the source structure;
2. understand the destination structure.
We can understand the source structure by inspecting the data in the browser console as we did previously.
I won't go into detail about how I arrived at this function; you can explore it yourself.
Search online for how to parse JavaScript objects, loop through them, and return new structures.
I will write another function to draw the line chart with our prepared data.
To recap, we used asynchronous code in JavaScript to fetch data from an API, THEN created a visual once the data were ready.
Let's store the returned data so we can use it as needed, without having to refetch the data each time.
Type az.hold_value.fetched_data into the console and hit enter.
We can access our covid-19 data anytime by simply working with this object.
To get our UI elements to do something we need to add "event listeners." These fire when a user interacts with our elements.
Let's hook up our events now.
First, we want to redraw the line chart when the user makes a choice from the dropdown. Let's use Azle's "change" event to make this happen.
Let's see if it works:
Adding events to our other elements works the same way. But those elements relate to calling some back-end model to make predictions using our covid-19 data.
So before adding the other events let's start working on the back-end model.
We could use a cloud provider such as Digital Ocean or Amazon, but since we're just prototyping ideas we'll use our own local computer.
This is what we need, since we want to give a back-end model data from our front-end and return a prediction.
At the beginning we created a my_app folder, currently holding our index.html file. Let's add another file to this folder called predict.py :
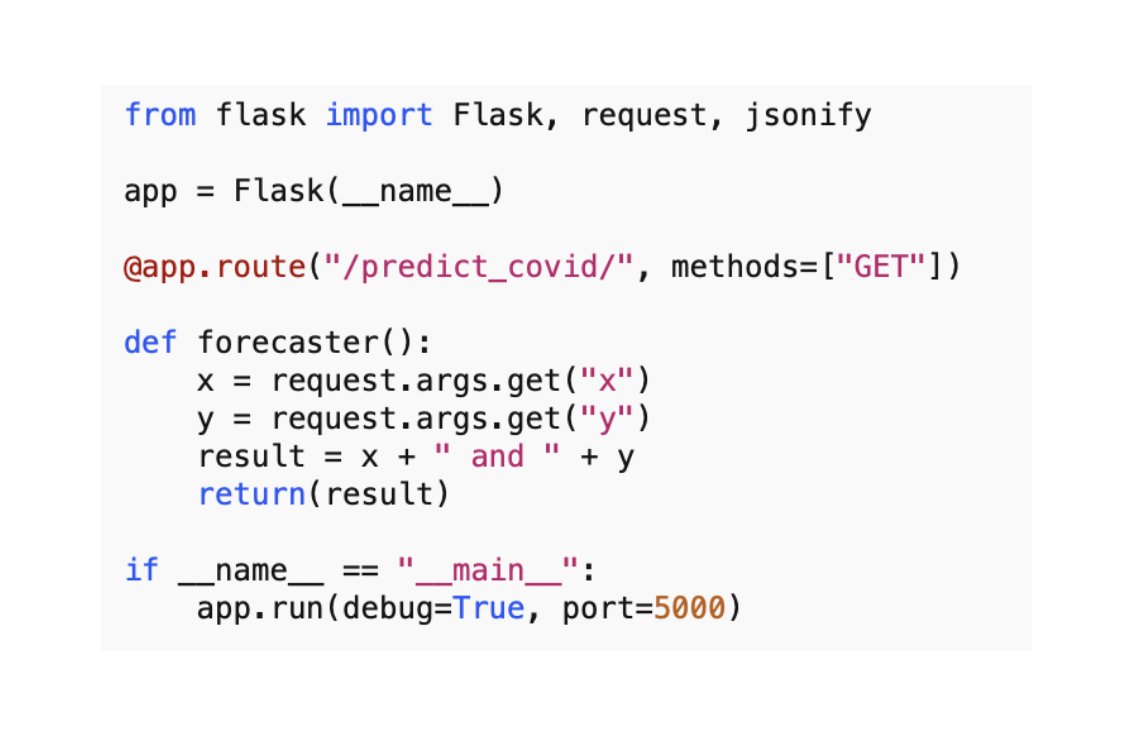
Above we are importing Flask, as well as the "request" and "jsonify" libraries, which will enable us to receive and send data from-and-to our front-end.
I called our function forecaster.
I chose port 5000. You can choose a different number if you want (if it's already being used your computer will tell you)
Open terminal. Quickest way in Mac is to use Spotlight Search by typing "command + spacebar" and typing in "terminal":
cd Desktop/my_app/
...and hit enter.
Now type:
ls
... and hit enter again.
Hard to believe our entire application is only 2 files. Thanks to the level of abstraction available in today's tools we only require minimal code to create a full blown app. 🙂
This is pretty cool, considering so many high-powered libraries are available today in Python. Just think what you could create! 🙃
To pass all this information "over the wire" we can construct the following URL:
http://localhost:5000/predict_covid/?x=100&y=400
If we succeed, the only thing left to do will be building a good forecasting model in Python.
- dropdown choice
- slider value
- dates and # of cases
Since we are only forecasting a few dates and values it makes more sense to use the data already fetched from the front-end.
We also added Azle's call_api inside the event's function property, specifying the URL to our Flask service, the "x" and "y" parameters, and an alert when data are returned.
Let's replace the "x" and "y" parameters with the actual data we need to send, and also add the forecast horizon from the slider value.
After some searching around I found a library called Prophet, which was open-sourced by Facebook.
facebook.github.io/prophet/
bit.ly/3d9ak36
You can look over the final predict.py file in the codebase on GitHub, which I link at the end of this thread.
I also added the new "draw_forecast" function to the "done" property of our call_api function.
Let's refresh our browser one last time to see the full application in action.
Before sending the code off to GitHub I want to make some final style changes.
I also want the Plotly chart to be transparent so we can see that nice blue background we had.
Finally, I'm going to change the title of our app now that it's something real.
github.com/sean-mcclure/G…