An async Django should look like a normal sync view but it runs asynchronously
"It takes them and runs them in equal time slices no matter what."
"By comparison, coroutines are cooperative."
They only yield when they hit an await.
"Cooperative is part of the name."
In some ways, they're safer than threads, but they're harder to think about.
"I can spin up an event loop and turn that sync thread into an async hosting thread."
"As amber alluded, async is fast as long as you're I/O-bound. Luckily we write websites, and websites are pretty much I/O-bound full time"
"You cannot have one function that you can call from a synchronous context and an async...
"Asynchrony is great, but it should be an optional add-on."
"I love Django. Django is a big framework... it is very stable and predictable.
"We cannot have cache.get()" be sync and async.
It creates double the maintenance burden, too.
There's a separate time.sleep() vs asyncio.sleep()
WSGI: you can run flask, django, or 'the awful little app I wrote in a weekend' on it
In the old days, "this framework had to use this web server"
I'd be interested...
It's more comms between client and server.
"We have to consider that async code is running in a thread and if you call async code...
Also have to consider backwards compat, which Django has tried to maintain since 1.0.
"And we have to keep that."
"Async has to add to Django. We can't replace it."
"Things should look familiar and still feel Django-ish."
"Django should stop you from shooting yourself in the foot... DEBUG is one exception for that"
Simplified block diagram time: handler, middleware, ORM, URL router, view, template, forms
Phase 1: talking to ASGI
Phase 3: async ORM
"These three each have their own benefit. The first phase, ASGI support, is maybe the least useful to you, the end dev"
Django 3.0 has phase 1 and will launch in December.
Phase 2: async middleware will "almost certainly" hit Django 3.1
Phase 3 is "the largest, most difficult" part and will hopefully hit 3.2
They can use lots of API async calls. Even if we only implement half the ORM, that's a huge perf boost
He joined back in the 0.96 days. "Just so you know, Django is a smug, arrogant framework that doesn't play nice with others... or at least, that's the impression you'd get from reading the rants." @ubernostrum
"We have custom middleware. For those of you who weren't around in Python 10 years ago ... there was this wonderful grand version of WSGI middleware"
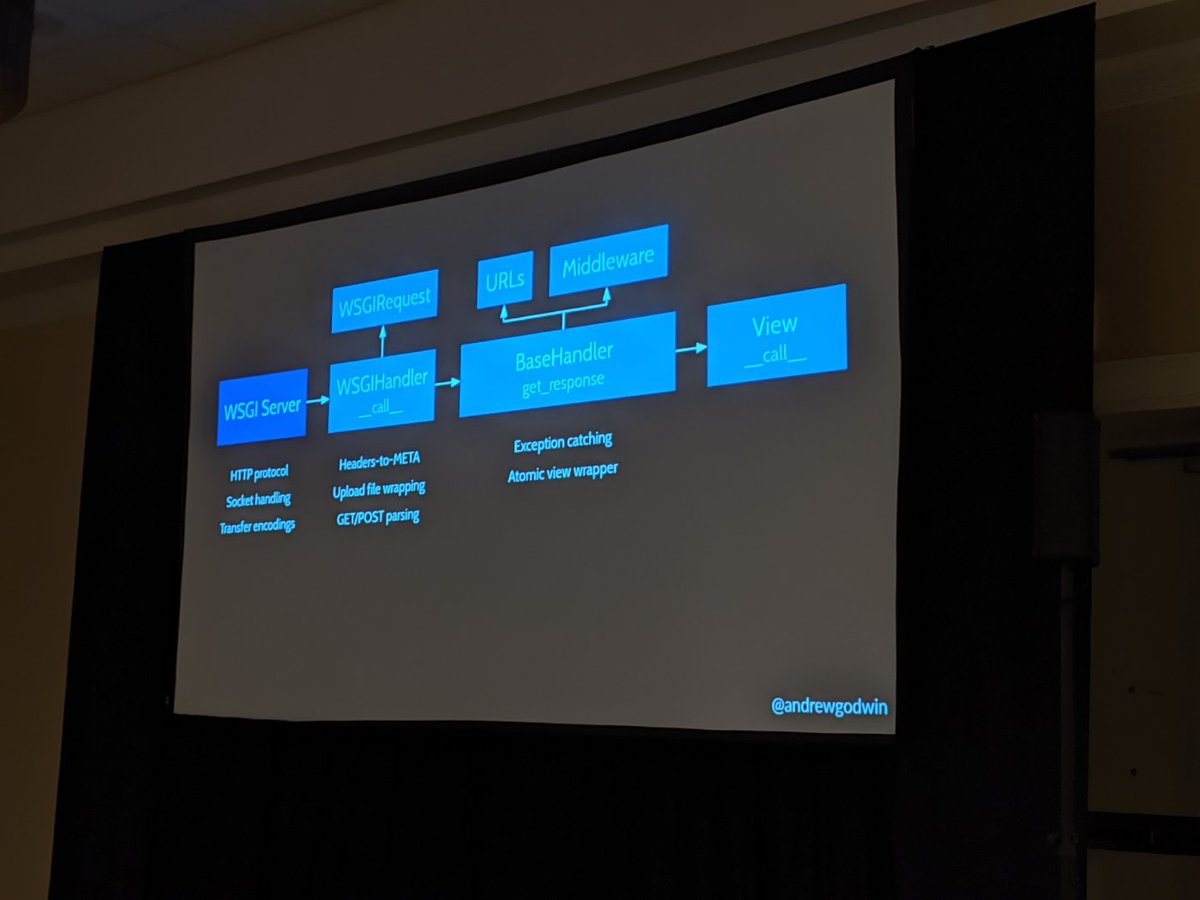
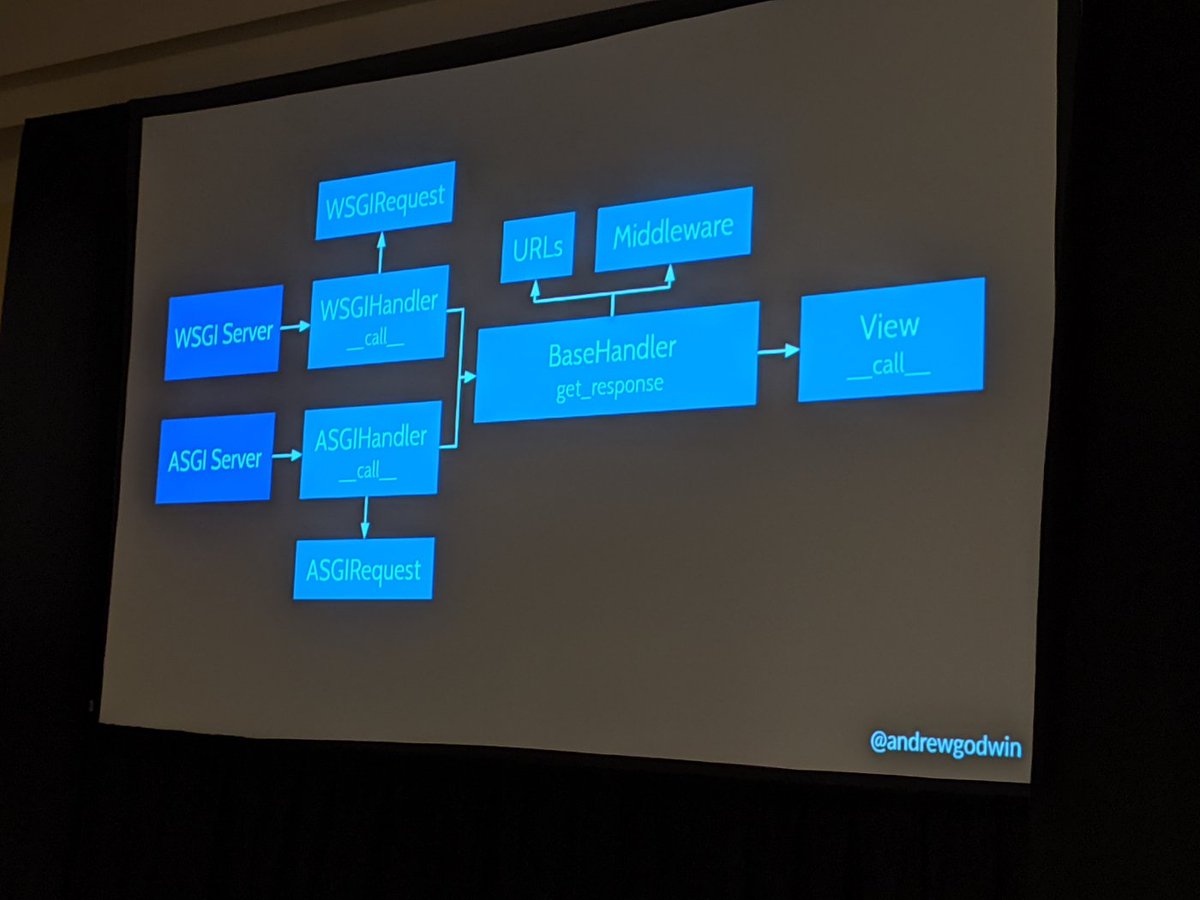
"One of the nice things as well is that ASGI is deliberately mostly WSGI-compatible"
"There are some tricky parts. Uploaded files are particularly fun"
"Thankfully, [calling async] is a danger you can understand and contain. What we have is a package called asgiref.sync.sync_to_async"
"Think about Andrew did it and others and they have tests and it's probably fine."
"The really good part is phase 2. I'm excited about this."
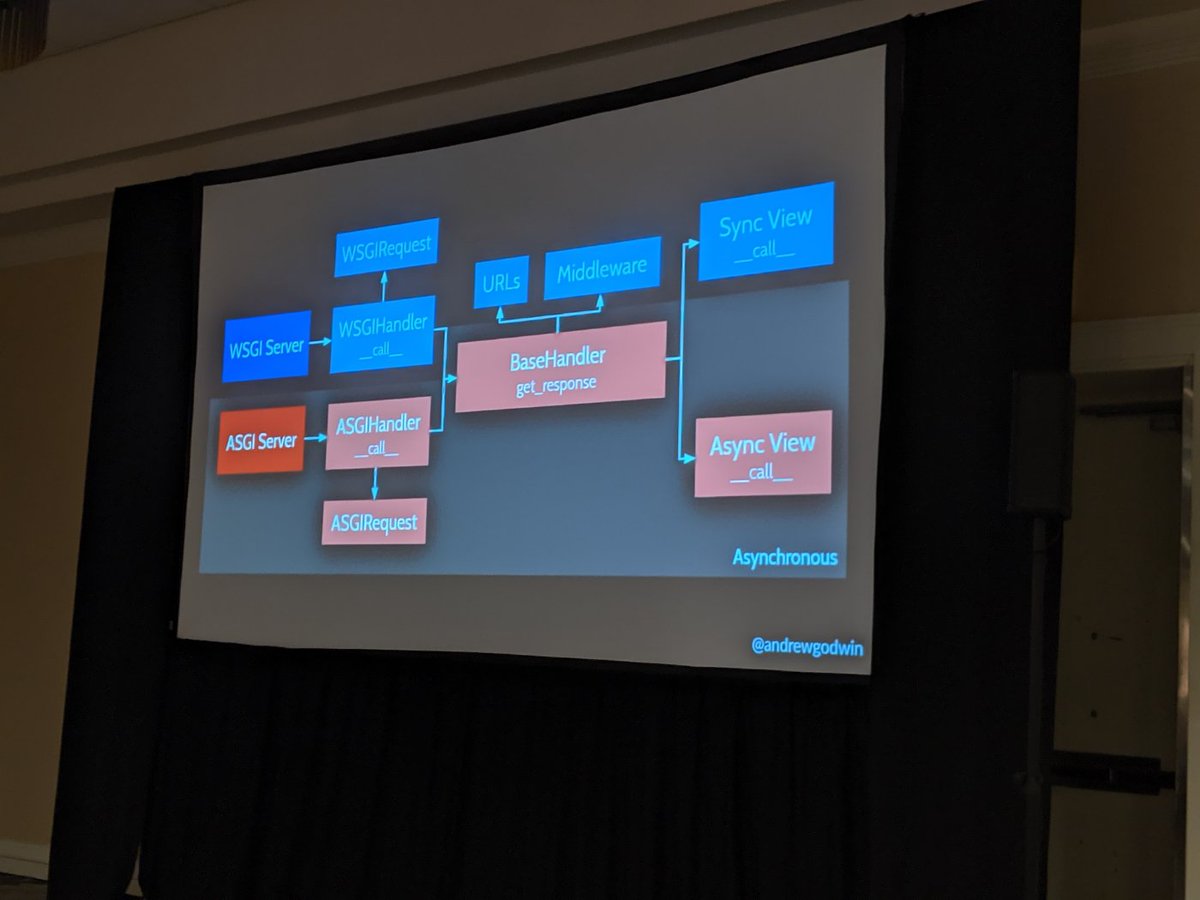
Also thanks to the test handler, "we are forever going to have sync code in the test client or handler calling into the new async base handler."
This means we have sync->async->sync, and "it leaves things in the request for things to do later blows up in the most spectacular fashion... I'm not going to tell you how I do it because it is awful."
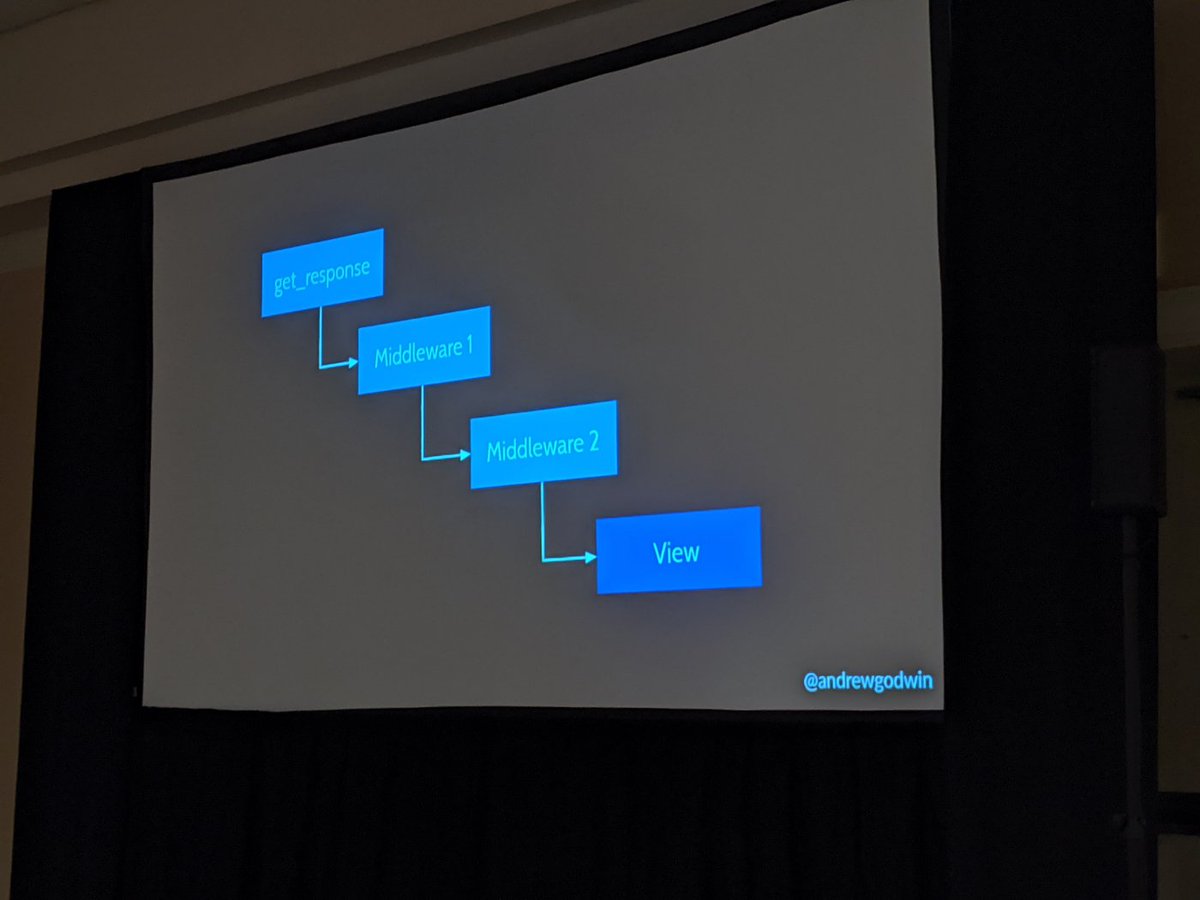
"We're sure the only around this is to write all of Django middleware to be natively sync"
"I have a branch where [async views] work and all but two tests pass, and that's why it's not in Django 3.0"
"Being Django-like is crucial. You have to have familiar but safe APIs."
"We can probably never have async traversing models, but you should probably use select_related() anyway."
"We did add one thing in Django 3, which is the ORM is fully aware of async safety."
It raises errors if you try to access the ORM in an async context.
"I've never worked on the query...
"Forms are perfectly fine" being sync.
"We have to be careful because performance is a concern."
TIL Django doesn't call...
Suggests auth middleware as a first-time contribution.
Calls for funding because async expertise is rare.
"The app magic removal was just when I entered Django."
"We haven't done one of these in a while... I need some way to talk to people... to have long conversations about weird async stuff."
"Surely, Andrew, async is just a flash int he pan"
"There are a lot of things that have been buzzwords or just a flash in the pan... we live in a world where everything we do is I/O bound. I can't think of more than one site I've worked...
"It is my personal believe that a well-written async Django app could get a 5-10x improvement if it's heavily I/O bound"
"Django is there to give you the ability to write beautiful amazing websites that people love and you don't put too much effort."
Slides are coming on twitter momentarily.
5 minute break before the next talk.