One Like, One Undefined Behavior.
I plan on running this through the weekend at least.
#Cplusplus
#Programming
int x1=std::numeric_limits<int>::max()+1;
int x2=std::numeric_limits<int>::min()-1;
[expr.pre]p4 eel.is/c++draft/expr#…
godbolt: godbolt.org/z/Ga-fbb

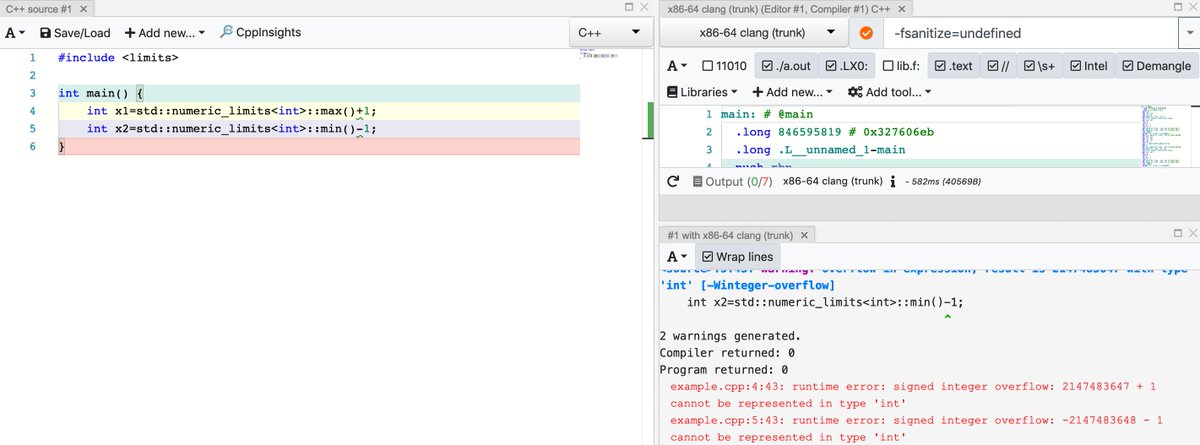
int x3=std::numeric_limits<int>::min() / -1;
[expr.pre]p4 eel.is/c++draft/expr#…
godbolt: godbolt.org/z/ytC8oi

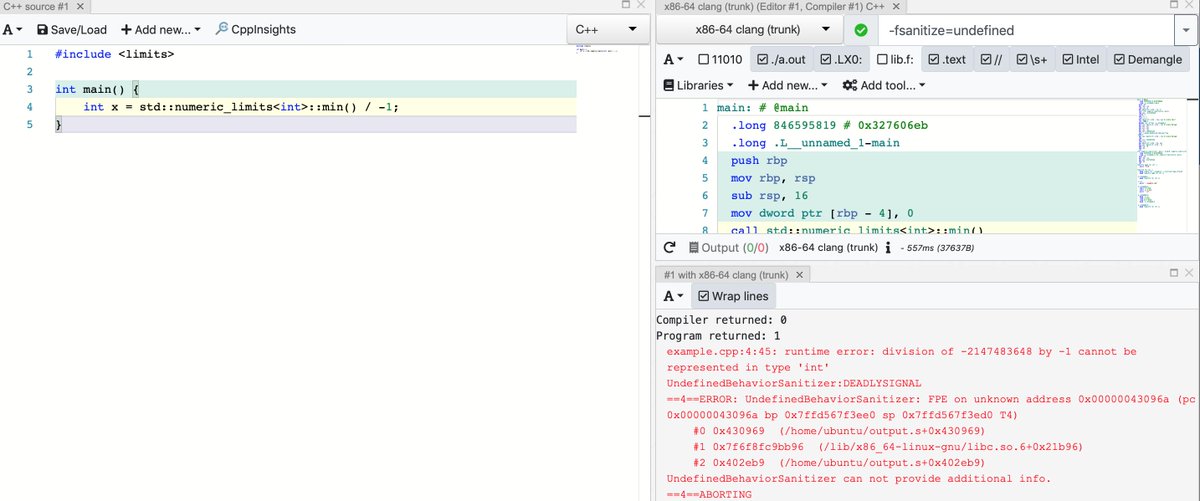
Gcc coalescing increment due to assumption of no undefined behavior
gcc turning a finite loop infinite due to the assumption of no undefined behavior
stackoverflow.com/a/24297811/170…
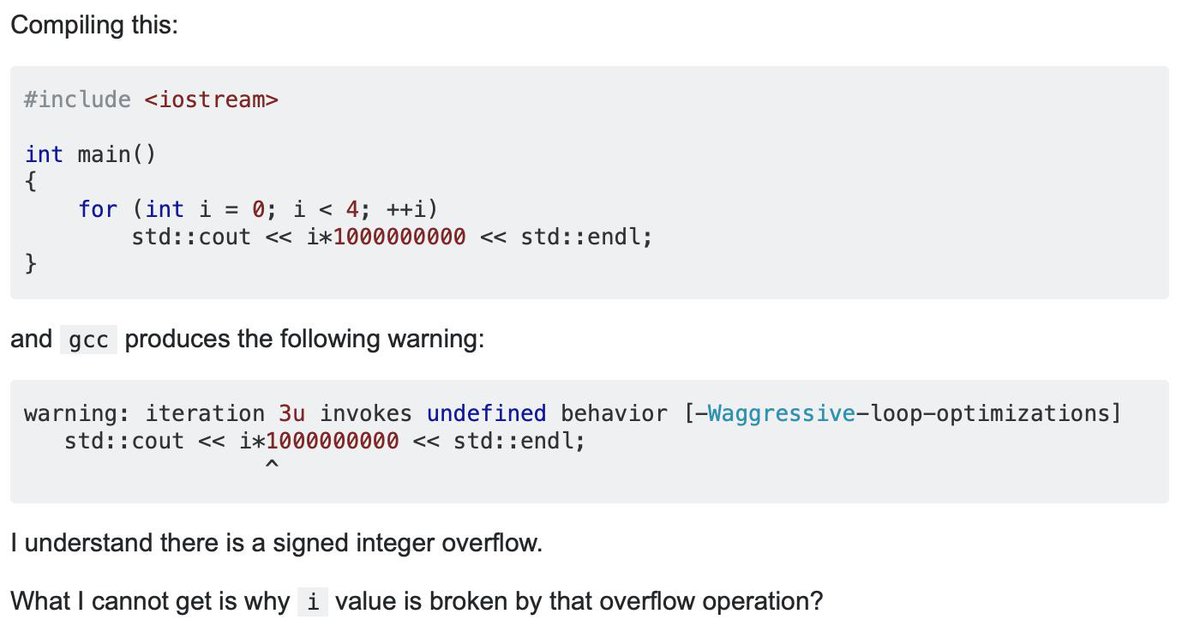
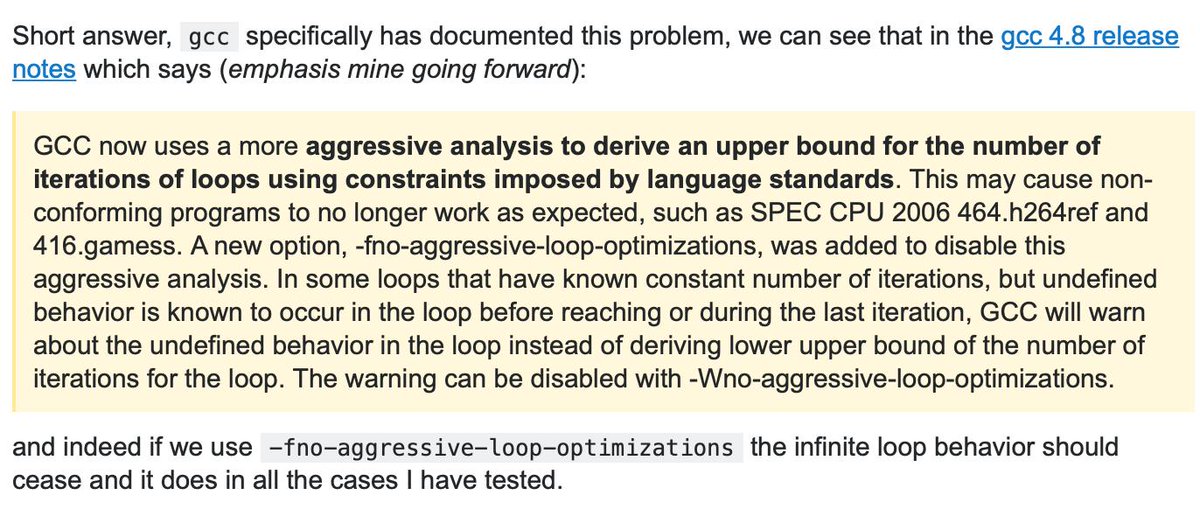
memcpy(dst, nullptr, 0);
C11 7.24.1p2 port70.net/~nsz/c/c11/n15…
Godbolt: godbolt.org/z/mxcHJY
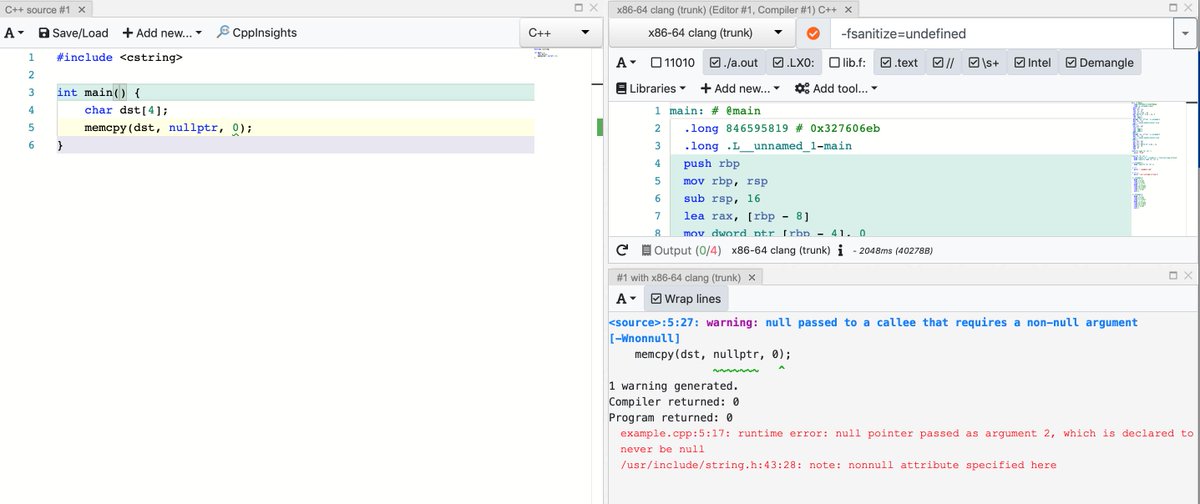

memcpy(arr, arr+1, 2);
C11 7.24.2.1p2 hhttps://port70.net/~nsz/c/c11/n1570.html#7.24.2.1p2
Godbolt: godbolt.org/z/dtUXqx

void f(size_t s) {
printf( "%d\n", s);
}
C11 7.29.2.1p9 port70.net/~nsz/c/c11/n15…
Godbolt: godbolt.org/z/Ee1VVm

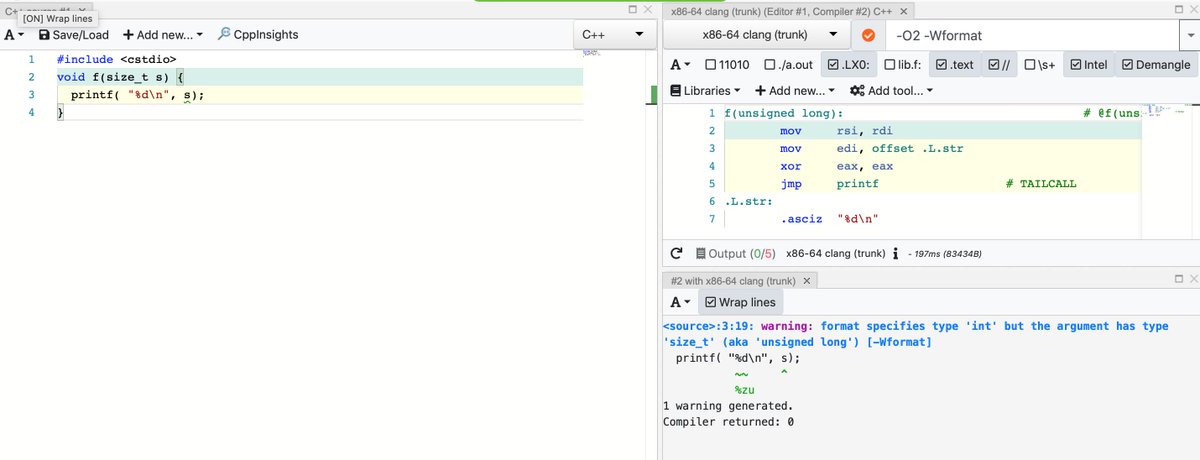
int f(int x) {
return x++ + x++;
}
[intro.execution]p10 eel.is/c++draft/basic…
Godbolt: godbolt.org/z/msejuQ
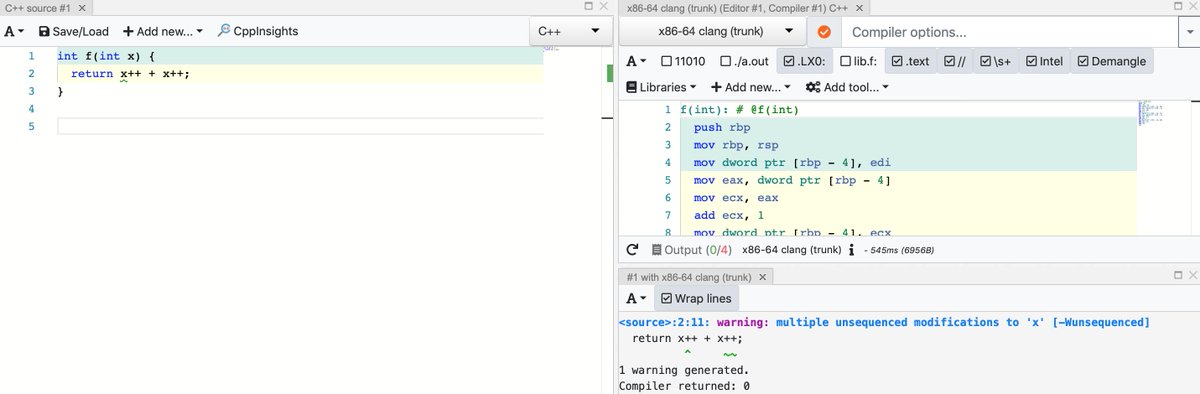
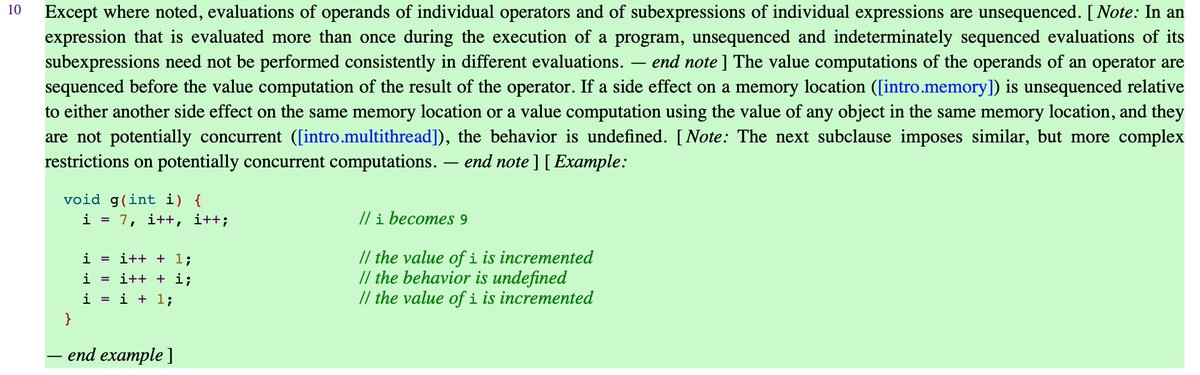
int cnt = 0;
auto f = [&]{cnt++;};
std::thread t1{f}, t2{f}, t3{f};
[intro.races]p21 eel.is/c++draft/intro…
Also see en.cppreference.com/w/cpp/language…
Godbolt: godbolt.org/z/0aktDl
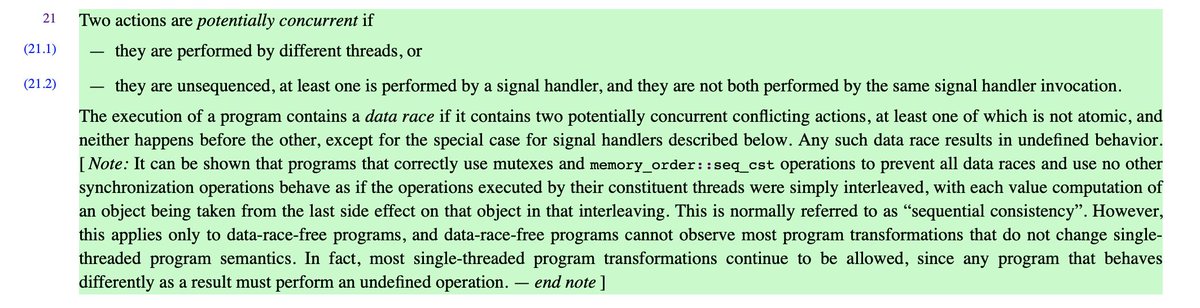
const char* p = "\\
u0041";
[lex.phases]/p2 eel.is/c++draft/lex.p…
godbolt: godbolt.org/z/xZLefc
DR787 open-std.org/jtc1/sc22/wg21…

#define GUARD_NAME ï ## _GUARD
[lex.phases]p4 eel.is/c++draft/lex.p…
godbolt godbolt.org/z/BO8PGi
N3881 open-std.org/jtc1/sc22/wg21…

#define S "
#define E "
int main() {puts(S hello world E);}
[lex.pptoken]p2 eel.is/c++draft/lex.p…

const char *p1 = "hello world\n";
char *p2 = const_cast<char*>(p1) ; // const_cast is already suspicious
p2[0] = 'm' ;
[lex.string]p15 eel.is/c++draft/lex.s…
godbolt: godbolt.org/z/wrNAzz

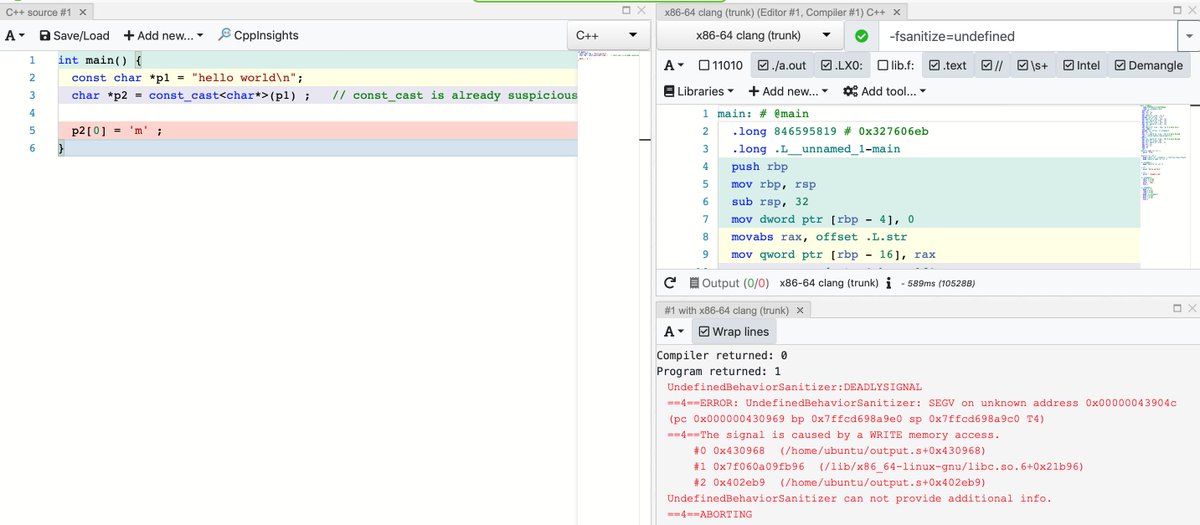
[basic.def.odr]p12 eel.is/c++draft/basic…
Examples from: DCL60-CPP. Obey the one-definition rule: wiki.sei.cmu.edu/confluence/dis…
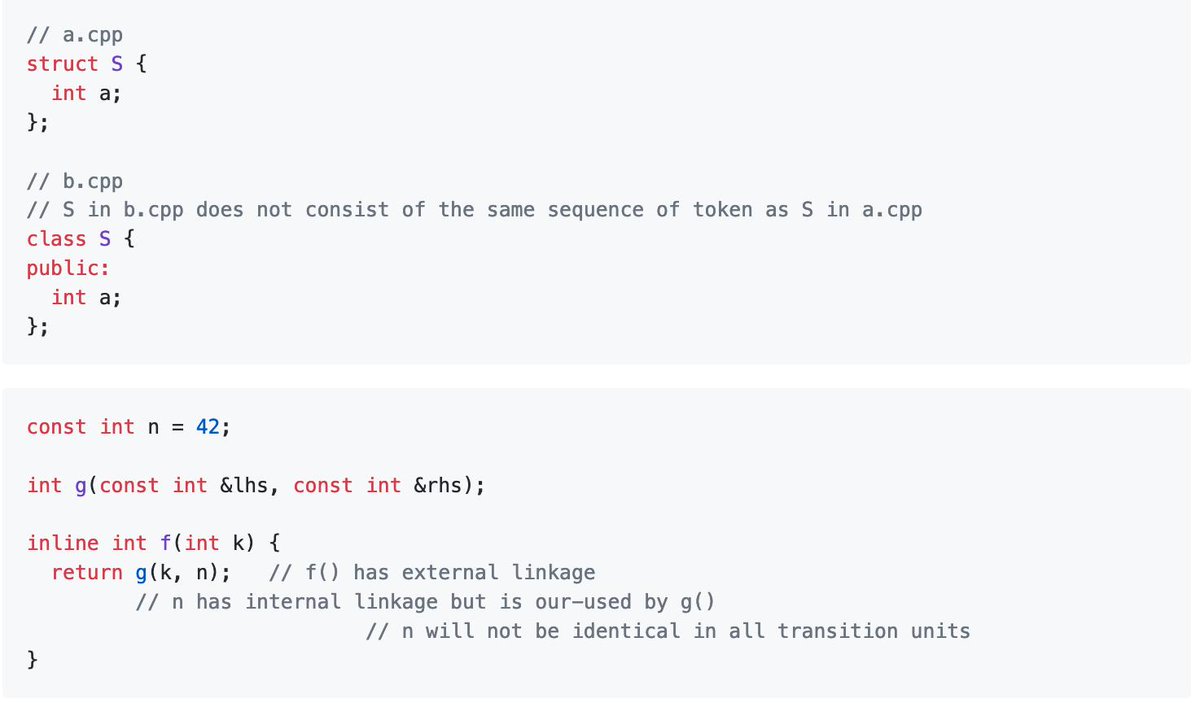
hubicka.blogspot.com/2014/09/devirt…
bool p;
if ( p )
puts("p is true");
if ( ! p )
puts("p is false");
[basic.indet]p2 eel.is/c++draft/basic…
Also see “Both true and false: a Zen moment with C” markshroyer.com/2012/06/c-both…
Also see:
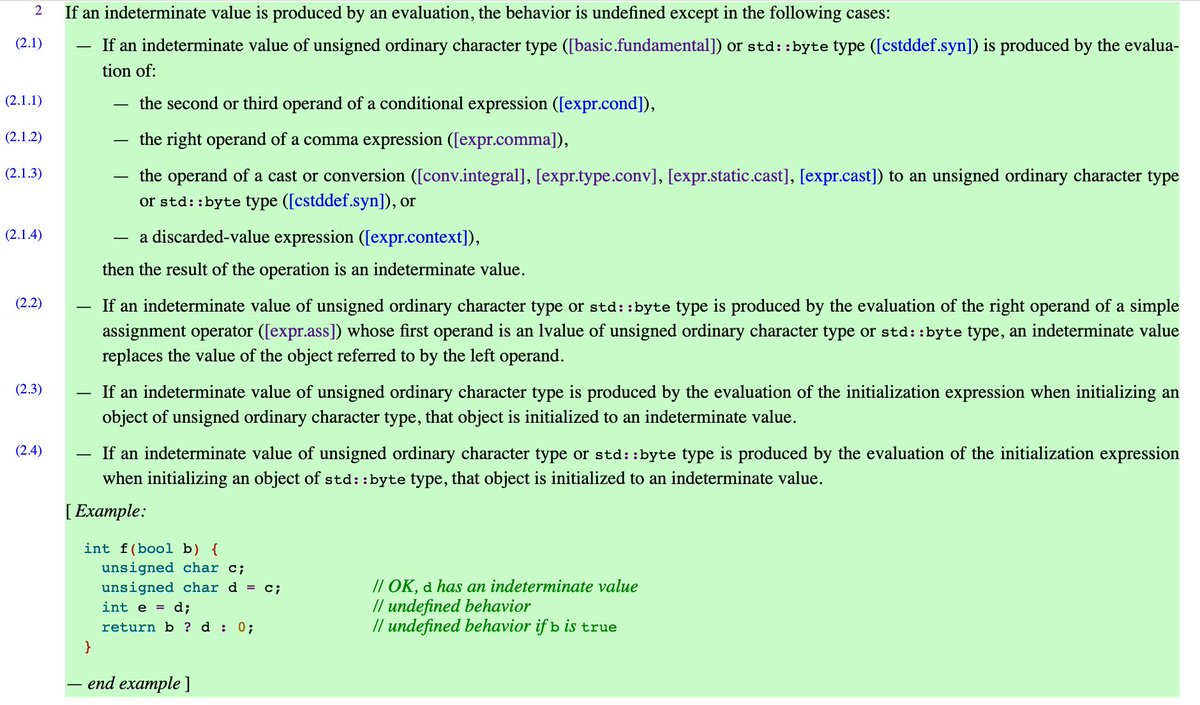
int main() {
decltype(main()) x;
return static_cast<bool>(&main);
}
[basic.start.main]p3 eel.is/c++draft/basic…
Godbolt: godbolt.org/z/VhCoop
Also see: stackoverflow.com/q/25297257/170…

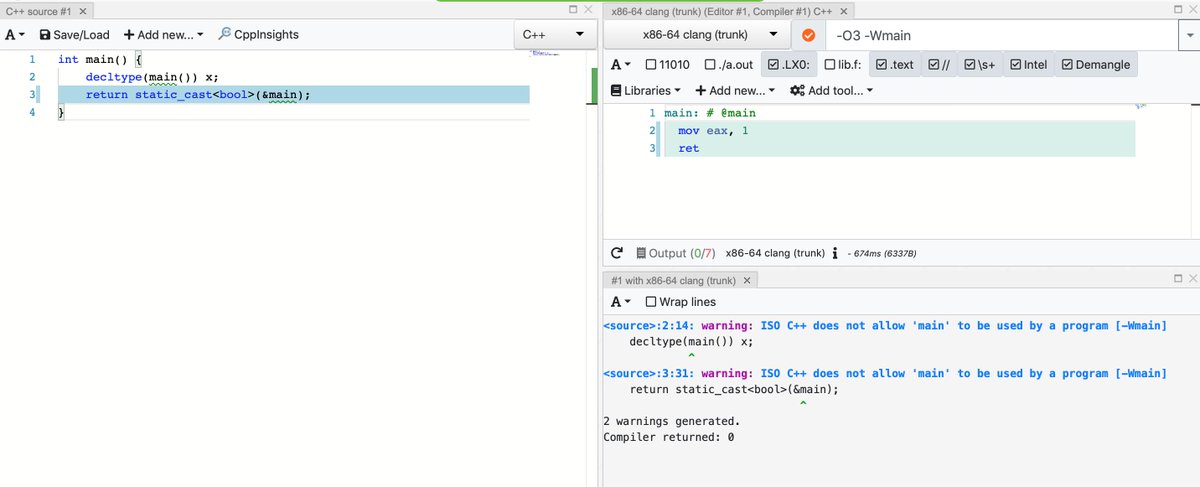
double d2=DBL_MAX;
float f=d2;
[conv.double]p1 timsong-cpp.github.io/cppwp/n4659/co…
godbolt: godbolt.org/z/1nHInC
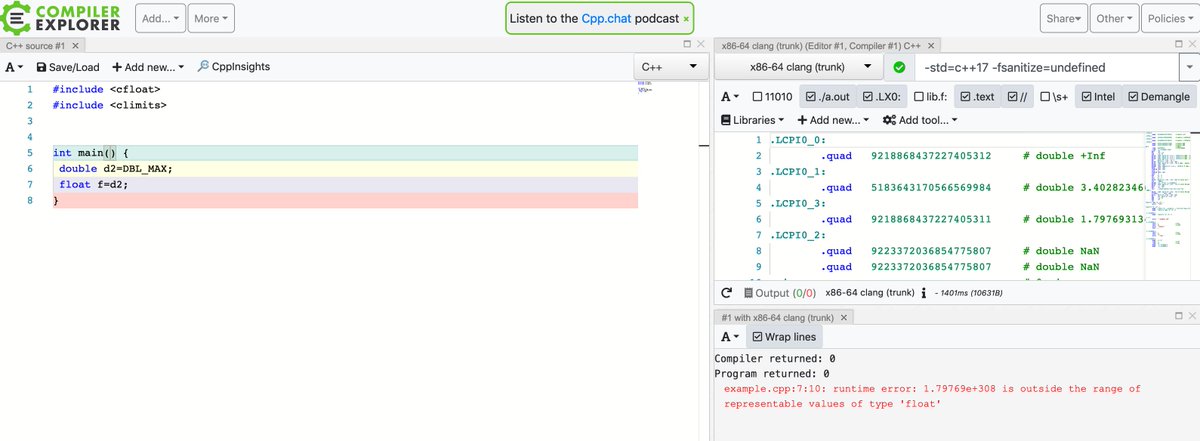

double d=(double)INT_MAX+1;
int x=d;
[conv.fpint]p1 timsong-cpp.github.io/cppwp/n4659/co…
godbolt: godbolt.org/z/Esp8ot
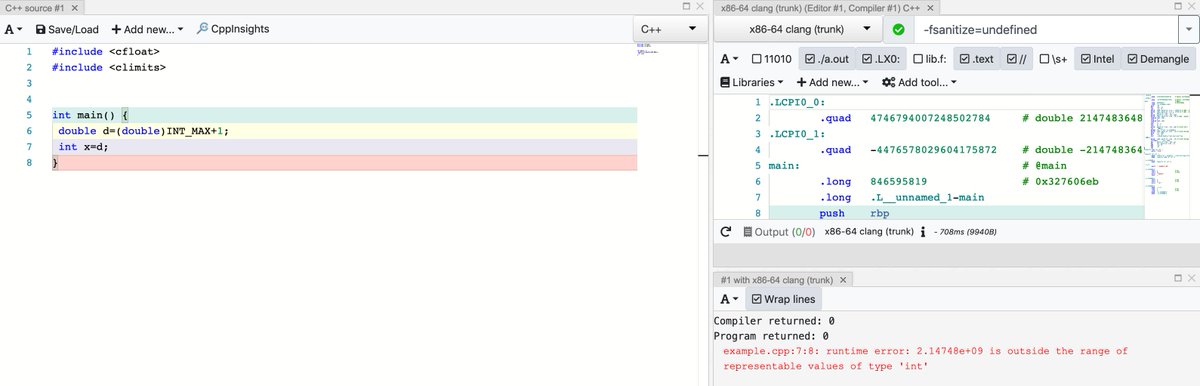

enum A {e1=1, e2};
void f() {
enum A a=static_cast<A>(4);
}
[expr.static.cast]p10 eel.is/c++draft/expr.…
and
[dcl.enum]p8 eel.is/c++draft/dcl.e…
godbolt: godbolt.org/z/csBeWj


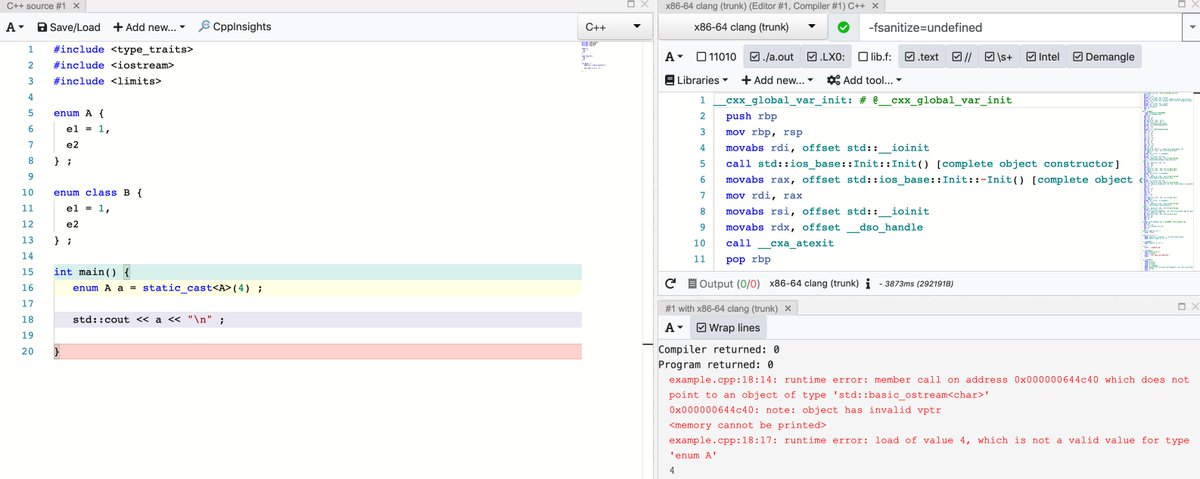
struct B {};
struct D1:B {};
struct D2:B {};
void f() {
B* bp = new D1;
static_cast<D2*>(bp);
}
[expr.static.cast]p11 eel.is/c++draft/expr.…
godbolt godbolt.org/z/ZU4cO4

int *x = new int;
delete [] x;
[expr.delete]p2 eel.is/c++draft/expr.…
godbolt: godbolt.org/z/6rOI4S

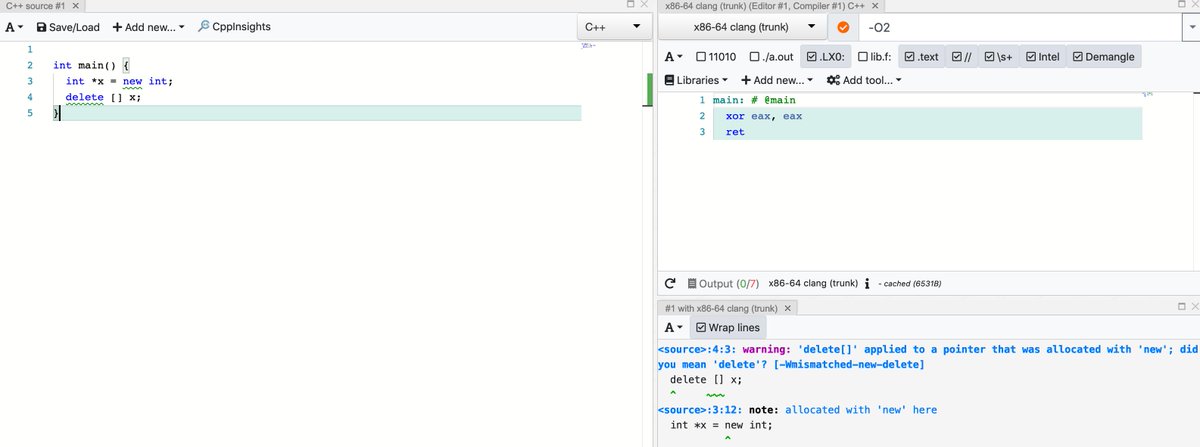
int *p = new int;
float *f = reinterpret_cast<float*>(p);
delete f;
[expr.delete]p3 eel.is/c++draft/expr.…
godbolt: godbolt.org/z/9AE_-F

struct A;
void f(A *p) {
delete p;
}
struct A {~A(){}};
[expr.delete]p5 eel.is/c++draft/expr.…
godbolt: godbolt.org/z/Jn_TFY
#cplusplus
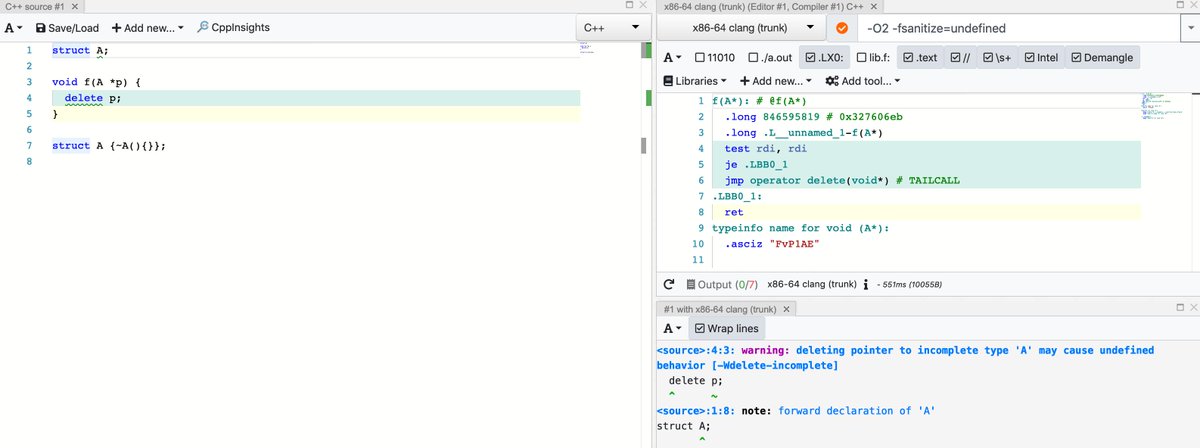

You can still do fun things w/ it though, at least in C:
const char main[] ="\xb8\x2a\x00\x00\x00\xc3";
See
Using an uninitialized int in a if statement and gcc and clang take different branches:
#cplusplus
int x = 1/0;
double d = 1.0/0.0;
[expr.mul]p4 eel.is/c++draft/expr.…
godbolt: godbolt.org/z/ayB189
#cplusplus
#programming

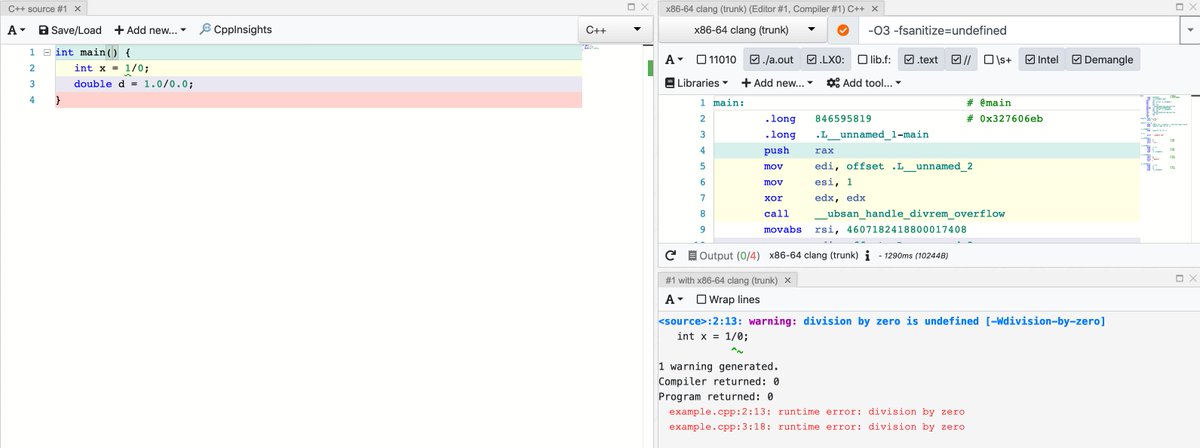
static const int arrs[10]{};
void f() {
const int* y = arrs + 11;
}
[expr.add]p4 eel.is/c++draft/expr.…
and
footnote eel.is/c++draft/expr.…
godbolt: godbolt.org/z/vFO-Qy
#cplusplus
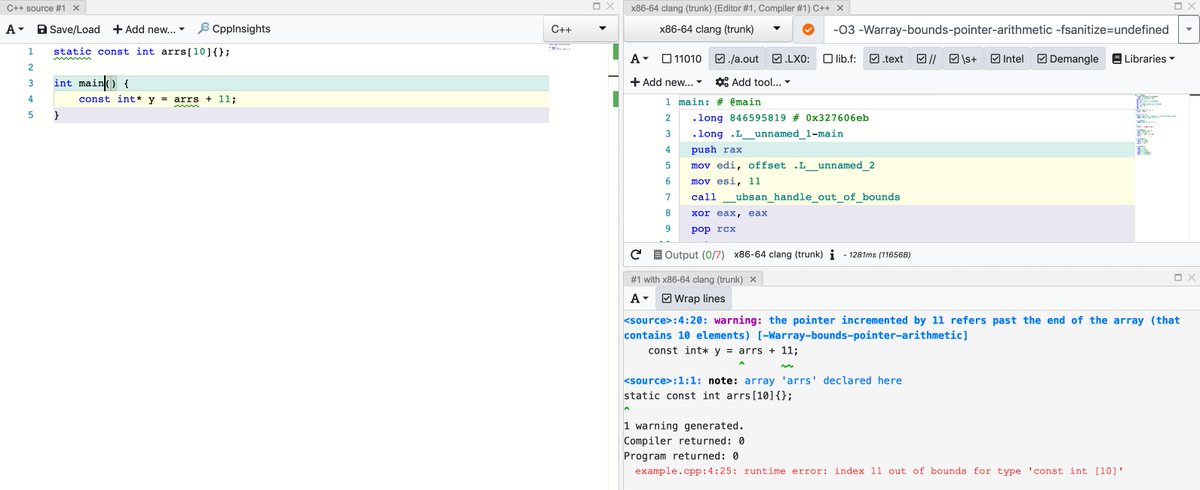


int x;
int y;
int *p1=&x;
int *p2=&y;
std::ptrdiff_t off = p1-p2;
[expr.add]p5.3 eel.is/c++draft/expr.…
godbolt: godbolt.org/z/JOMylq

char s1, s2, s3, s4;
strcpy(&s1, "str");
int y = 1 << -1;
[expr.shift]p1 eel.is/c++draft/expr.…
godbolt: godbolt.org/z/XPaWXE

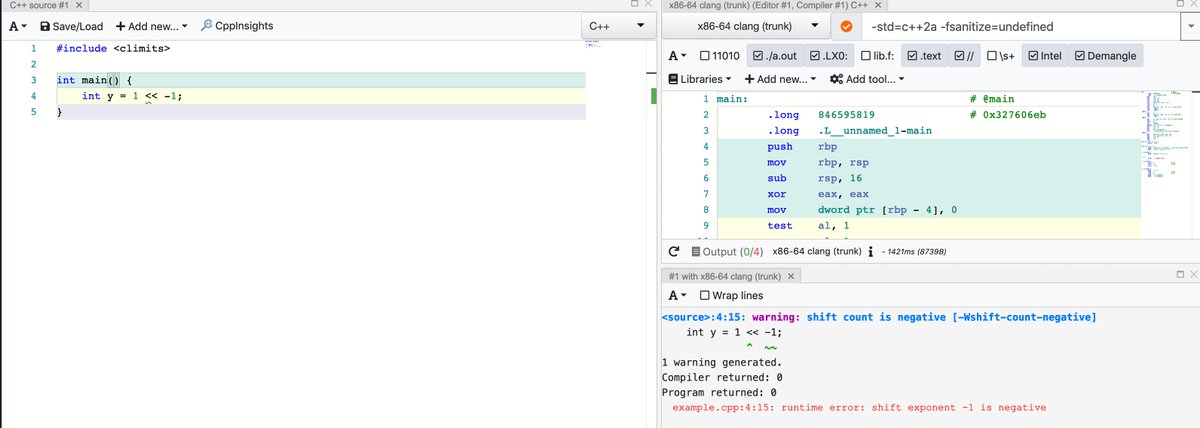
I still have a bunch of material, I did not realize how much those final touches for each one required :-p
static_assert(sizeof(int) == 4 && CHAR_BIT == 8 );
int y1 = 1 << 32;
int y2 = 1 >> 32;
[expr.shift]p1 eel.is/c++draft/expr.…
godbolt: godbolt.org/z/75TAvs
#cplusplus

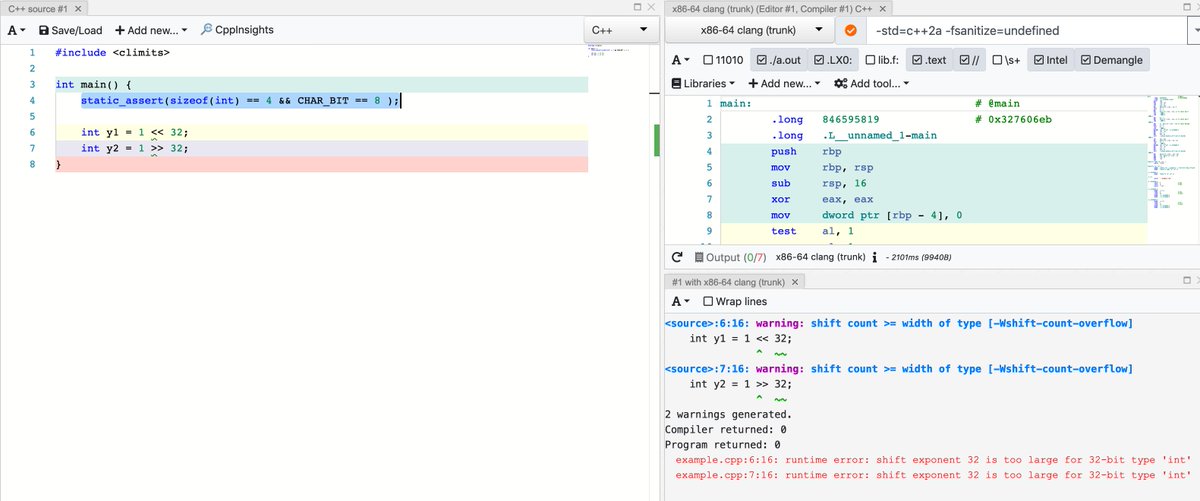
int y4 = -1 << 12;
[expr.shift]p2 timsong-cpp.github.io/cppwp/n4659/ex…
godbolt: godbolt.org/z/dlfgK5

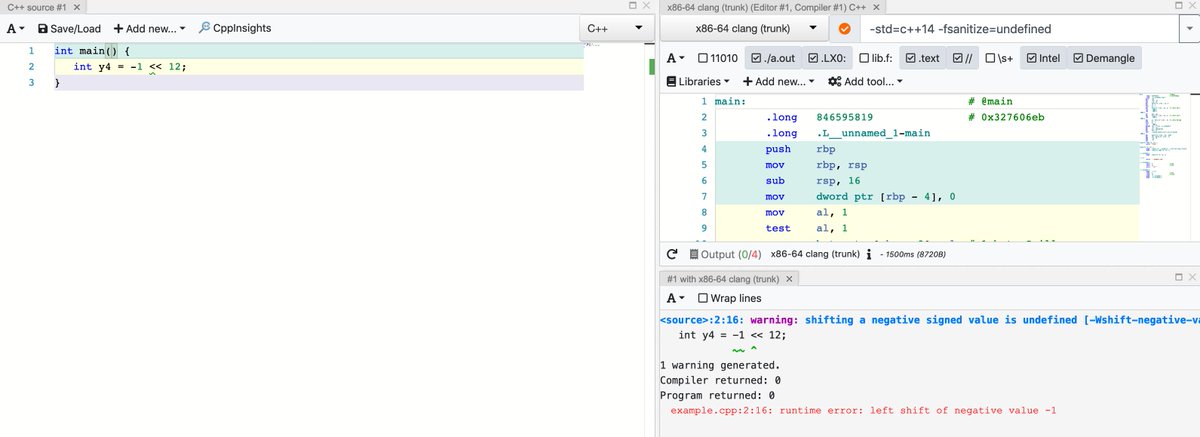
int x=1;
char *c=reinterpret_cast<char*>(&x);
x = *c;
[expr.ass]p8 eel.is/c++draft/expr.…
godbolt: godbolt.org/z/w9dnST
#cplusplus

int f(int x) {
if(x)
return 1;
}
void b(){
int x=f(0);
}
[stmt.return]p2 eel.is/c++draft/stmt.…
godbolt: godbolt.org/z/xAybG4
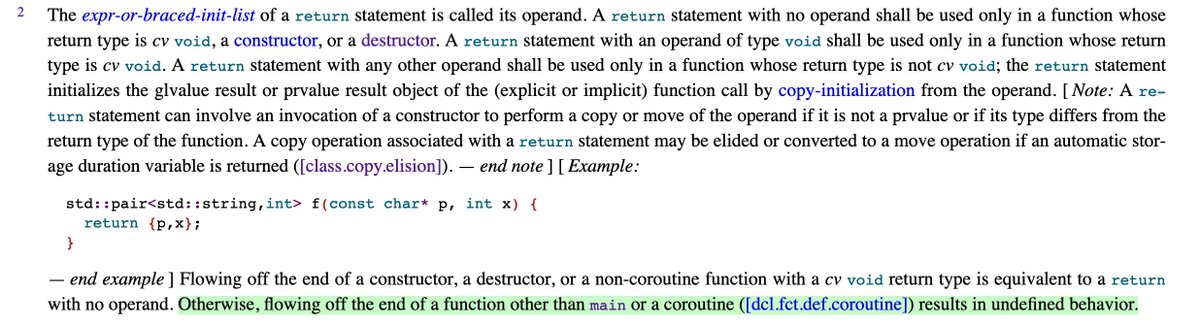
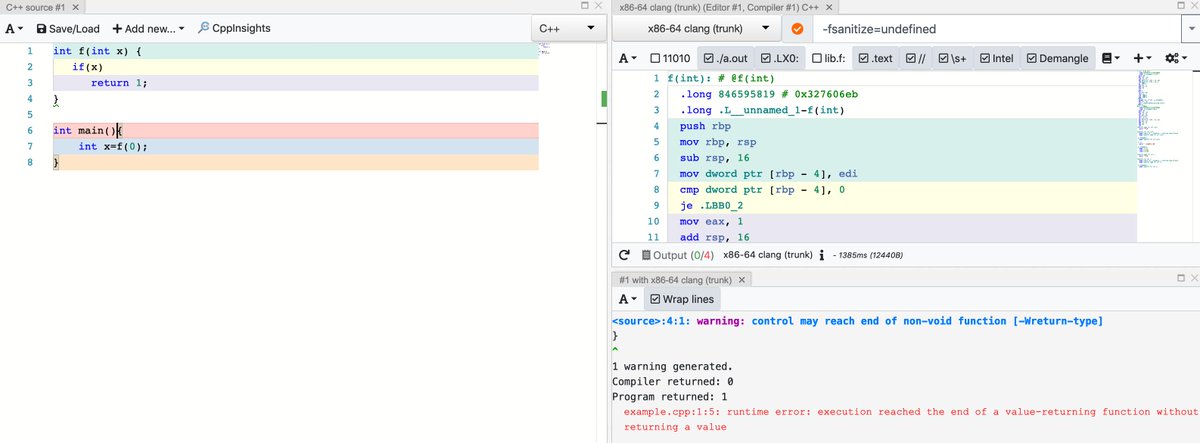
In this case it turn a loop infinite:
#cplusplus
clang and gcc optimizing differently in C and C++ for this since in C it is only UB if you use the value:
int foo(int i) {
static int s = foo(2*i);
return i+1;
}
[stmt.dcl]p4 eel.is/c++draft/stmt.…
godbolt: godbolt.org/z/MpQT_l
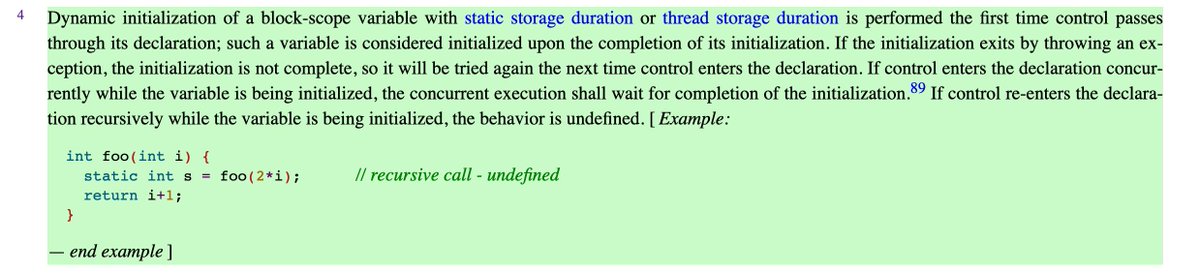
int b() {
const int x=1;
int *p = const_cast<int*>(&x);
*p = 2;
return *p;
}
[dcl.type.cv]p4 eel.is/c++draft/dcl.t…
godbolt: godbolt.org/z/p4YBux
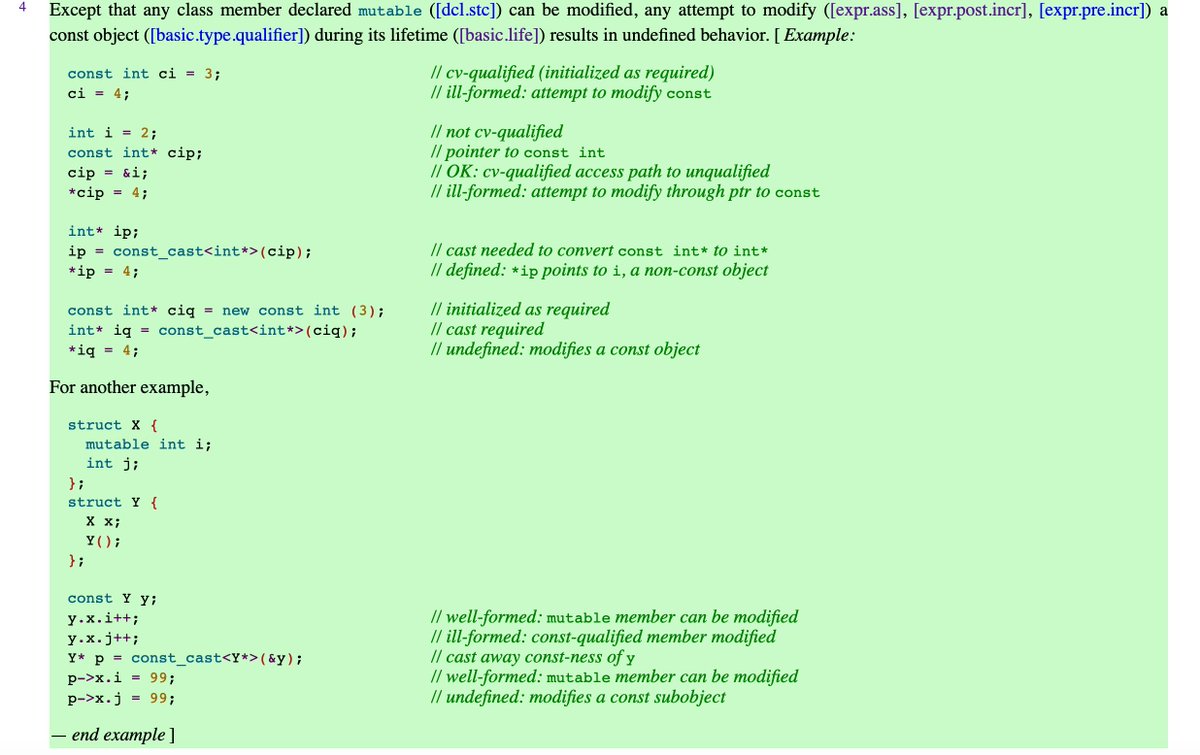
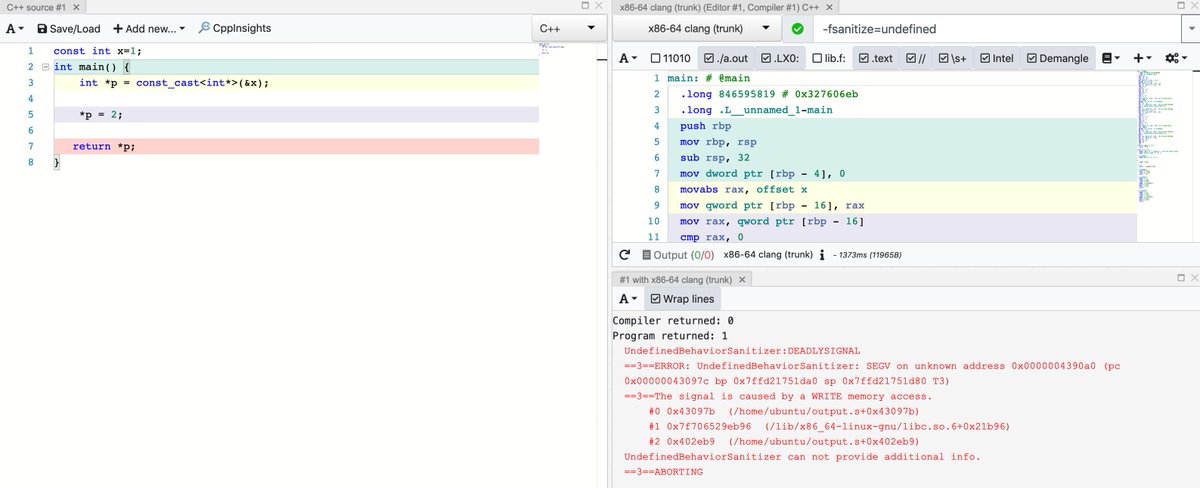
void f() {
volatile int x=0;
int &y=const_cast<int&>(x);
std::cout << y;
}
[dcl.type.cv]p5 eel.is/c++draft/dcl.s…
godbolt: godbolt.org/z/4xKsxy

void f(int x) [[expects audit: x>=1 && x<=2]];
void b() {
f(100);
}
[dcl.attr.contract.check]p4 eel.is/c++draft/dcl.a…
int f(int x)
[[ensures r: r == x]]
{
return ++x; // UB
}
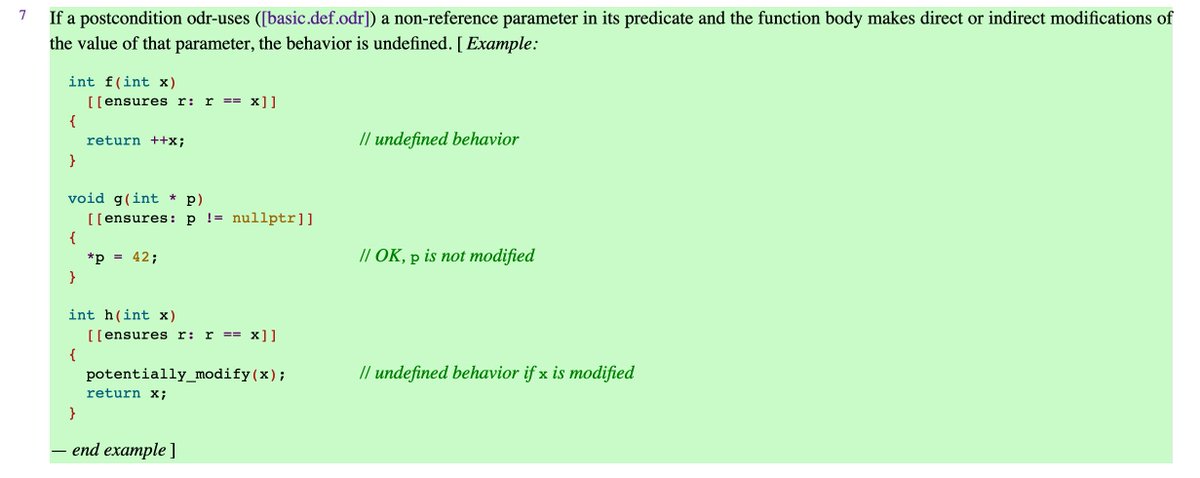
[[ noreturn ]] void q(int i) {
// behavior is undefined if called with an argument <= 0
if (i > 0)
throw "positive";
}
[dcl.attr.noreturn]p2 eel.is/c++draft/dcl.a…
godbolt godbolt.org/z/OZbnfb

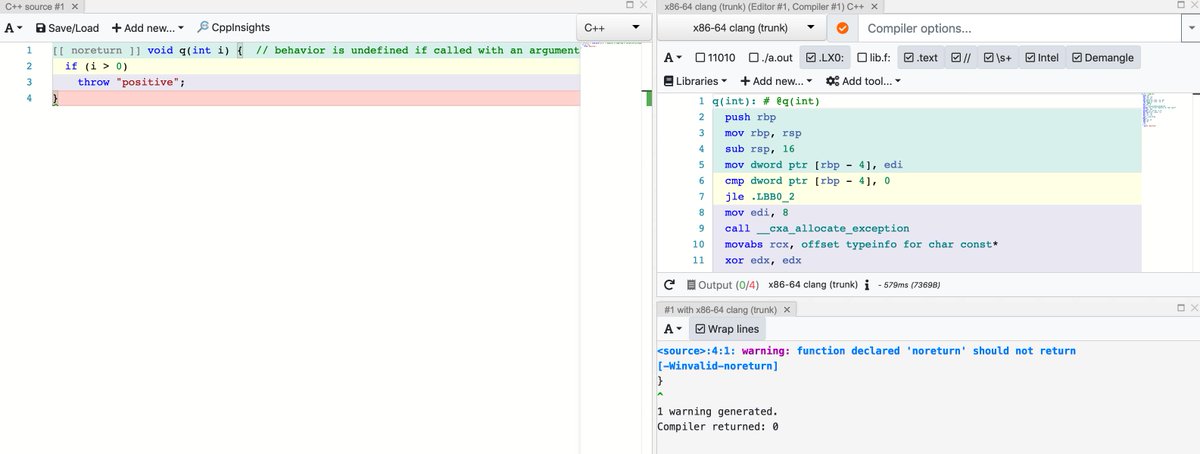
struct X {};
void f() {
X *x=nullptr;
x->~X();
}
[class.dtor]p14 eel.is/c++draft/class…
godbolt: godbolt.org/z/LWmK4f
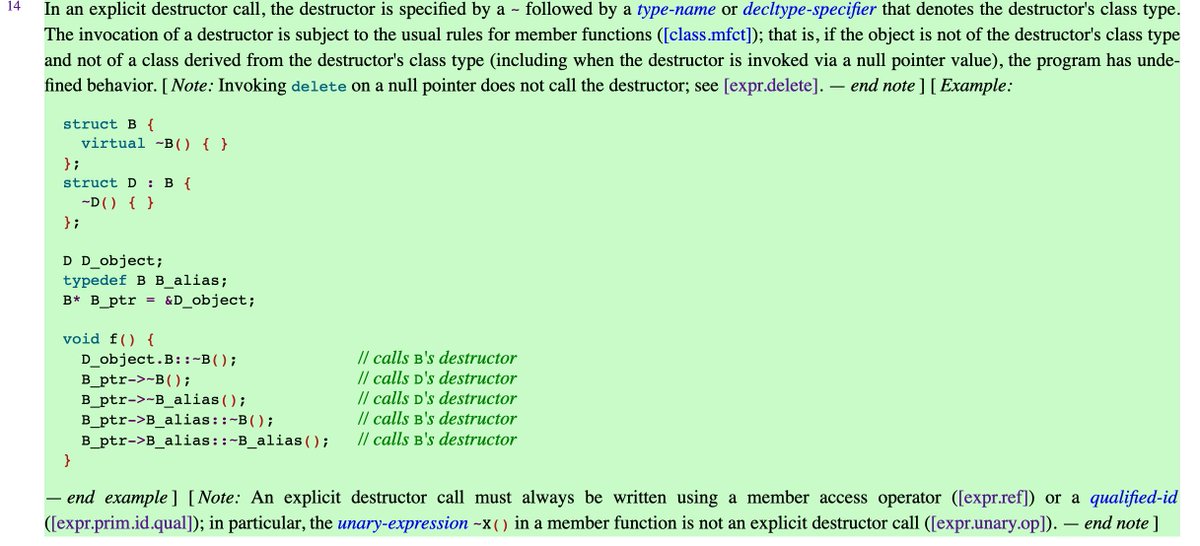
struct A{
~A(){}
};
int main() {
A a;
a.~A(); // Destructor will be invoked again at scope exit invoking UB
}
[class.dtor]p16 eel.is/c++draft/class…
godbolt godbolt.org/z/NeuBge

union Y { float f; int k; };
void g() {
Y y = { 1.0f };
// OK, y.f is active union member (10.3)
int n = y.k;
}
[class.union]p1 eel.is/c++draft/class…
godbolt: godbolt.org/z/oW1pnx

while(1)
;
[intro.progress]p1 eel.is/c++draft/intro…
Also see N1528: Why undefined behavior for infinite loops?: open-std.org/jtc1/sc22/wg14…

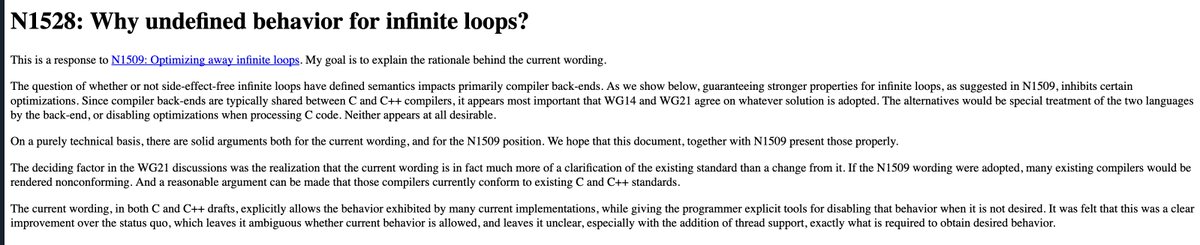
int* p = nullptr;
*p=0;
CWG active issue 232: open-std.org/jtc1/sc22/wg21…
CWG closed issue 315: open-std.org/jtc1/sc22/wg21…
Godbolt: godbolt.org/z/hnT_oV
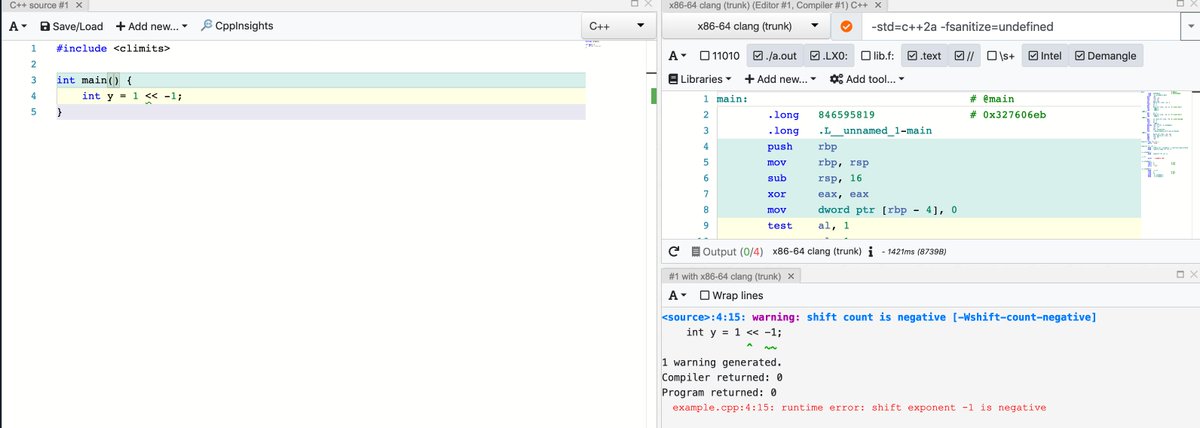
struct A{
int g(){return 0;}
static int f(){return 1;}
};
A* a=nullptr;
void f() {
int x = a->f();
int y = a->g();
}
CWG 315: open-std.org/jtc1/sc22/wg21…
Godbolt godbolt.org/z/WxpKae
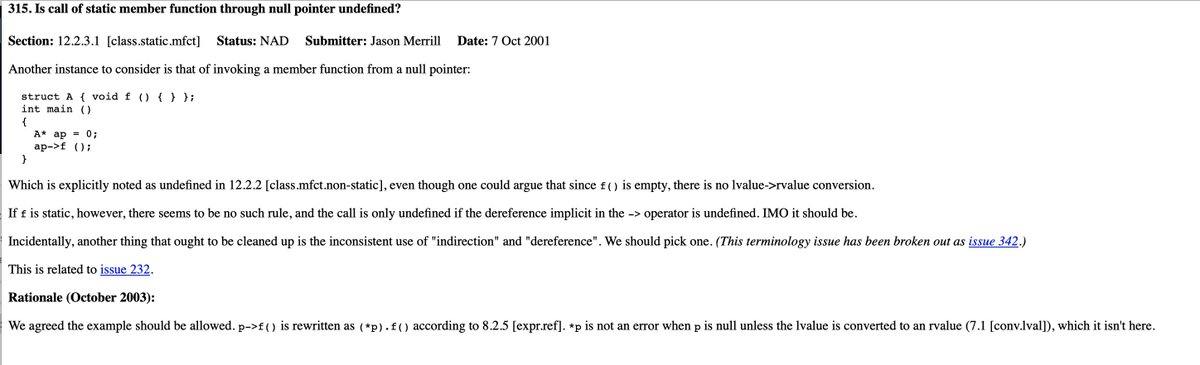
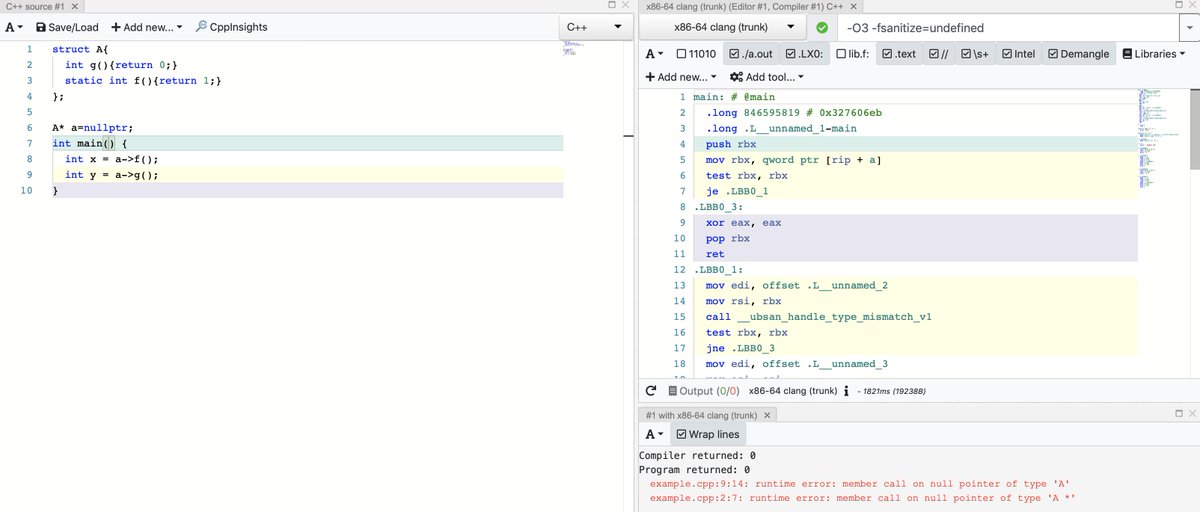
auto g(int a, int b, int c)
{
std::initializer_list<int> v = {a,b,c};
return v;
}
DR 1299 open-std.org/jtc1/sc22/wg21…
Issue 1565 open-std.org/jtc1/sc22/wg21…
Godbolt using -Wlifetime: godbolt.org/z/W25e1R
#cplusplus
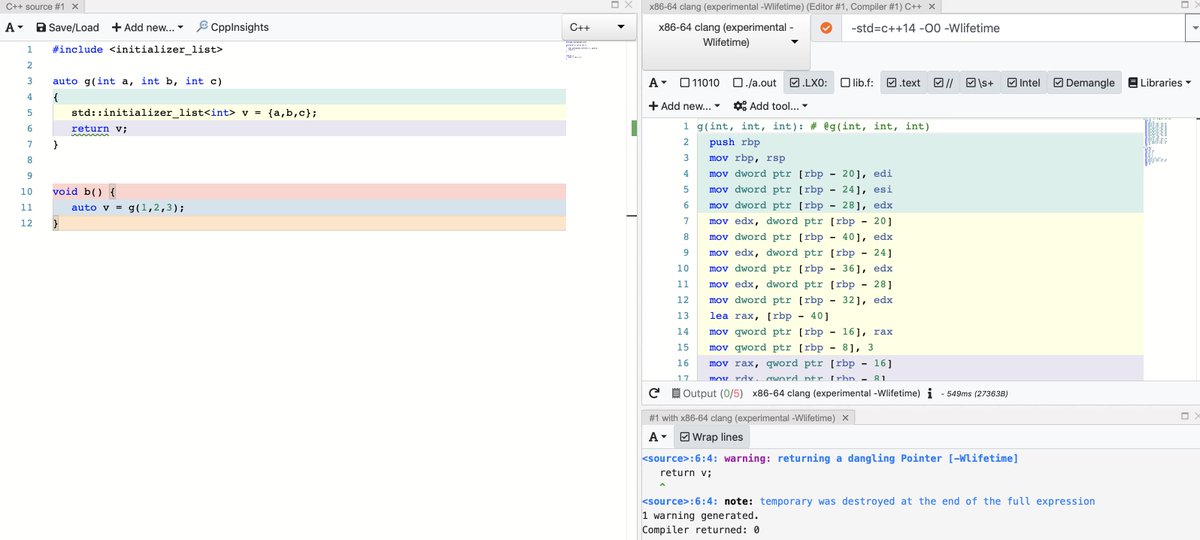
auto f(){
int x=0;
auto f=[&](){return x;};
return f;
}
[basic.life]p1.5 eel.is/c++draft/basic…
[basic.stc.auto]p1 eel.is/c++draft/basic…
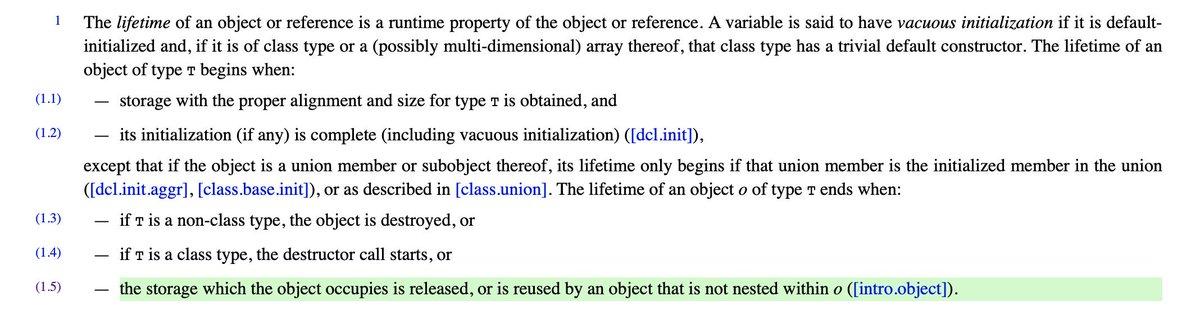

Oh look at that out of bounds counting seems to have struck 😱
int arr[10]{};
int x = arr[20];
[expr.add]p4 eel.is/c++draft/expr.…
godbolt: godbolt.org/z/H2XAko
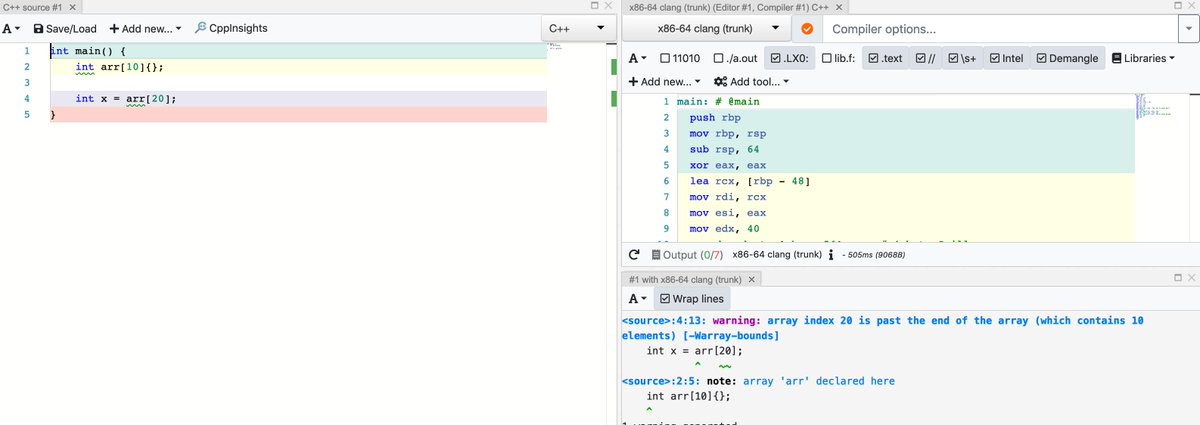
complex<int> mc[4] = {0};
for(int di = 0; di < 4; di++, delta = mc[di]) {
cout << di << endl;
}
See: stackoverflow.com/q/32506643/170…
Another example: stackoverflow.com/q/24296571/170…
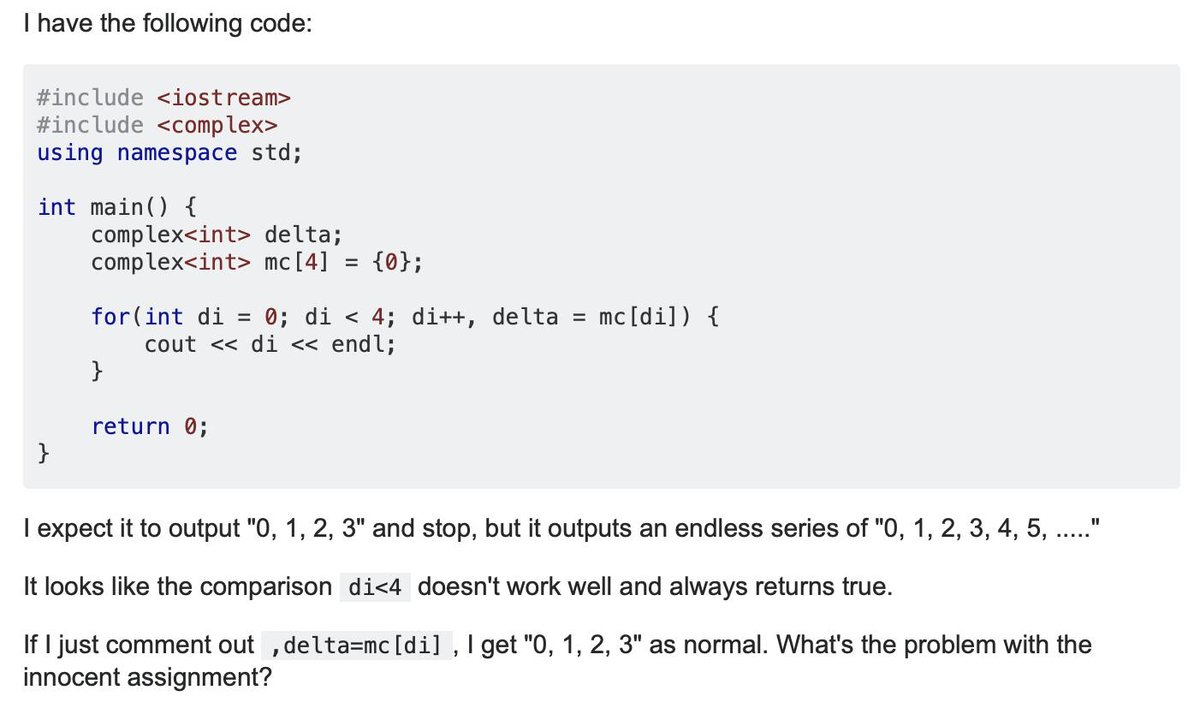
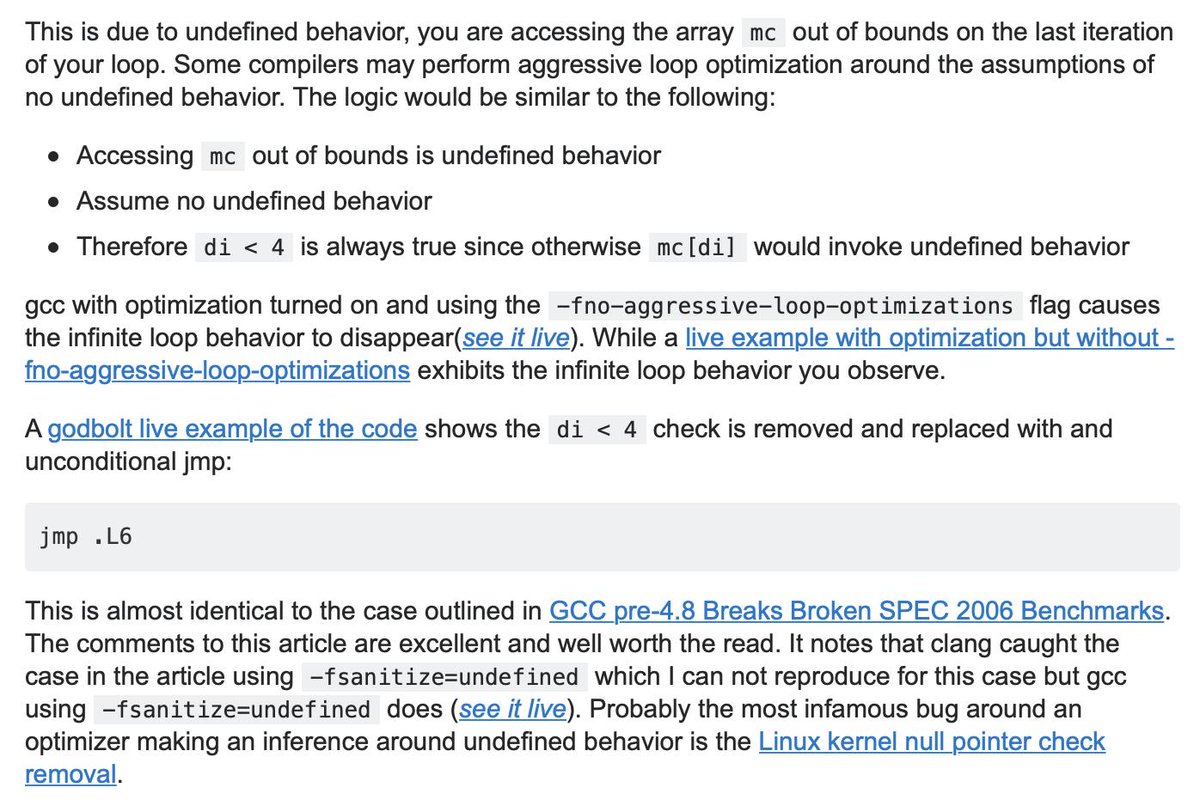
int *x = new int[0];
*x = 0;
[basic.stc.dynamic.allocation]p2 eel.is/c++draft/basic…
Godbolt: godbolt.org/z/-8fh5U
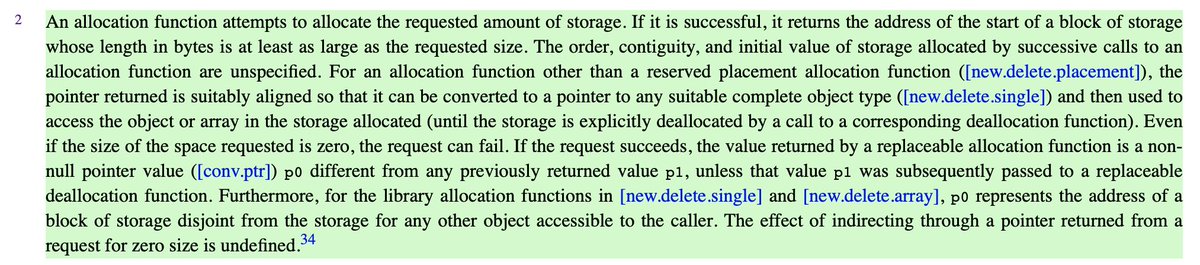
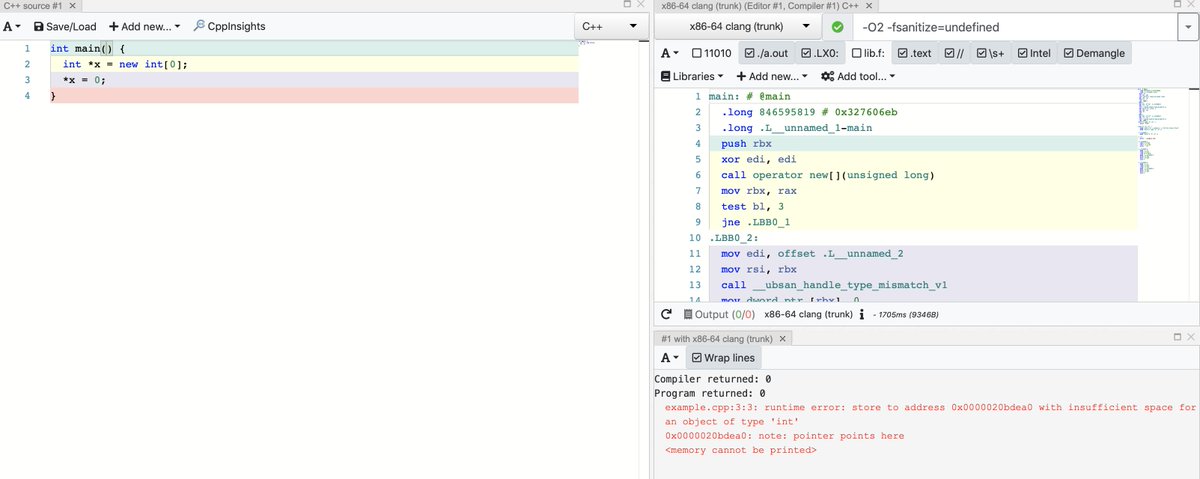
uint16_t x = std::numeric_limits<uint16_t>::max();
uint16_t y = std::numeric_limits<uint16_t>::max();
auto z = x*y;
[conv.prom] eel.is/c++draft/conv.…
godbolt: godbolt.org/z/jwBlnc
h/t @Myriachan
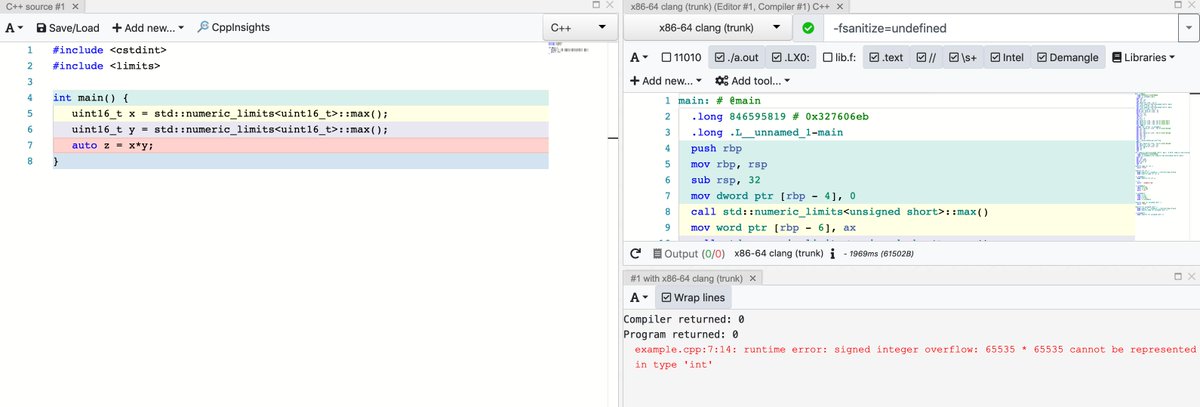
