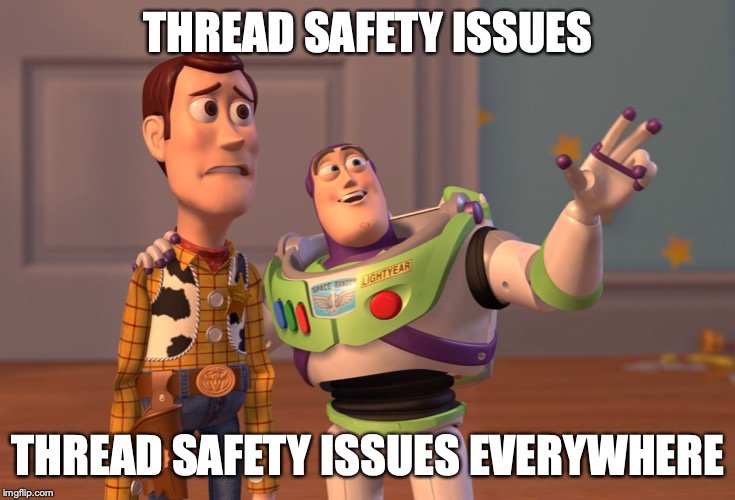
Unfortunately, most folks don't have time to do that, so let's talk about what thread safety crashes look like on Apple OSes.
1. SIGSEGV on near-NULL ptr (0x10, 0x20).
2. SIGSEGV on valid-ish heap address.
3. SIGABRT (NSException) for -doesNotRecognizeSelector:.
4. SIGSEGV on "random"/garbage address.
CoreFoundation: __NSFastEnumerationMutationHandler
libsqlite3.dylib: sqlite3MutexMisuseAssert
stackoverflow.com/questions/3139…