In this thread I'm going to show how I create, update and invoke lambda functions in 1 to 2 seconds (which makes a massive difference in the OODA and REPL lambda dev workflow)
The code below (which was executed in 4s) created the lambda by:
- zipping current project code
- uploading zip file to s3
- creating lambda function
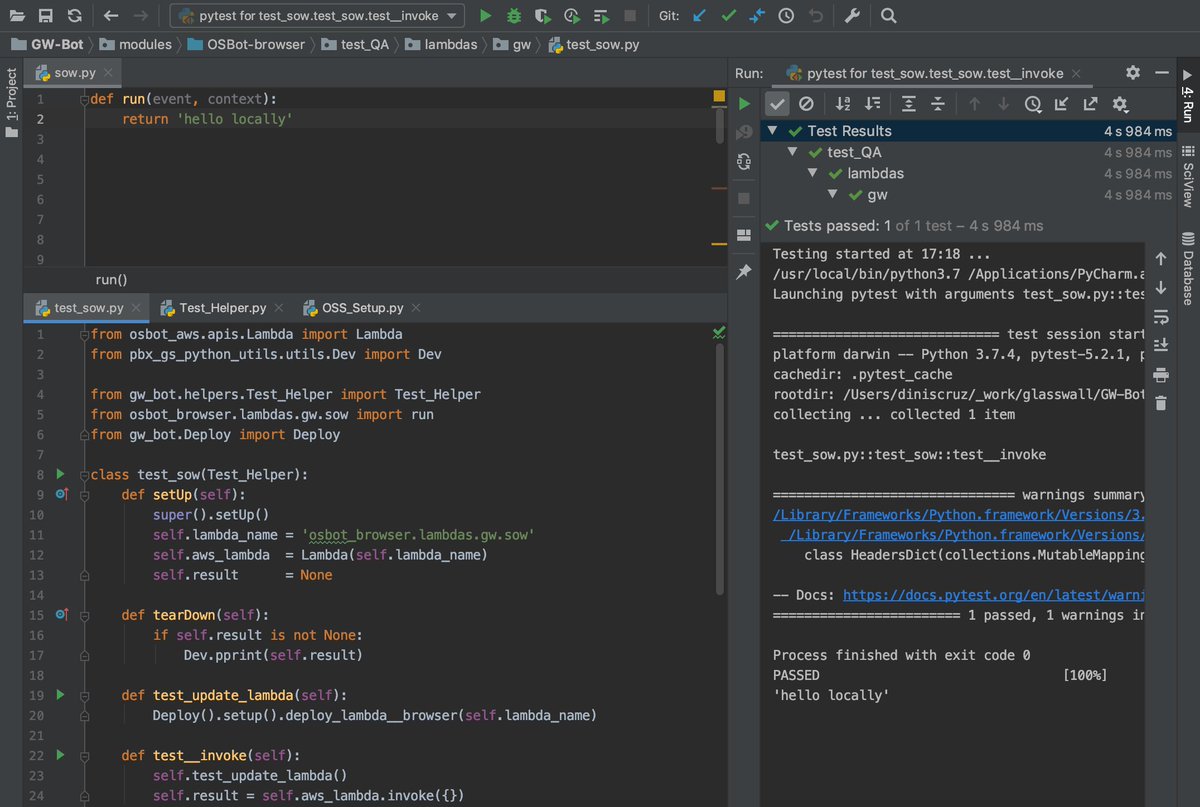
- the return value from the lambda function was changed
using the test:
- the deploy_lambda__browser function will:
a) re zip code
b) upload zip to s3 and
c) reconfigure lambda
- invoke the lambda function
all in 2.5 secs :)
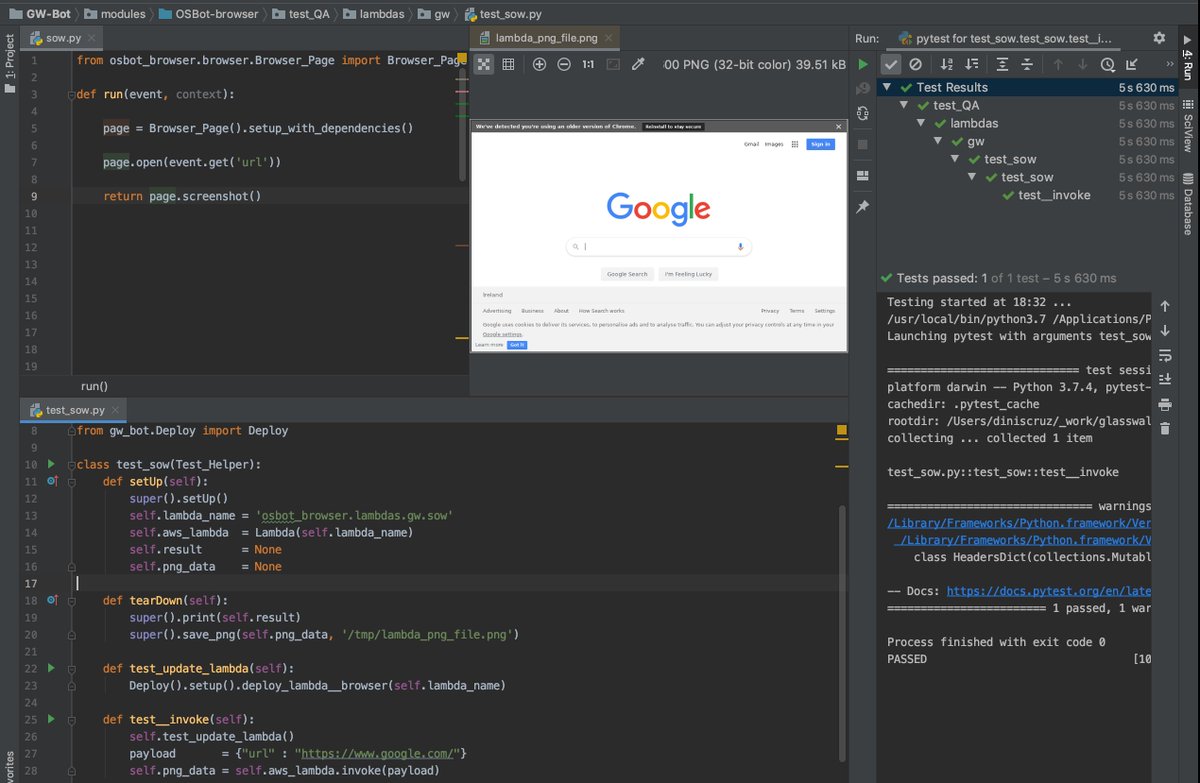
Let's see this in action
THAT is very powerful, since it enables all sorts of amazing workflows: logging in, feeding data to a page, interacting with pages, navigate, and fixing UIs
The ability to take screenshots from a locally running webserver
Since lambda is basically just a linux box running python, it is very easy to start a local webserver
(see commit github.com/filetrust/OSBo…)
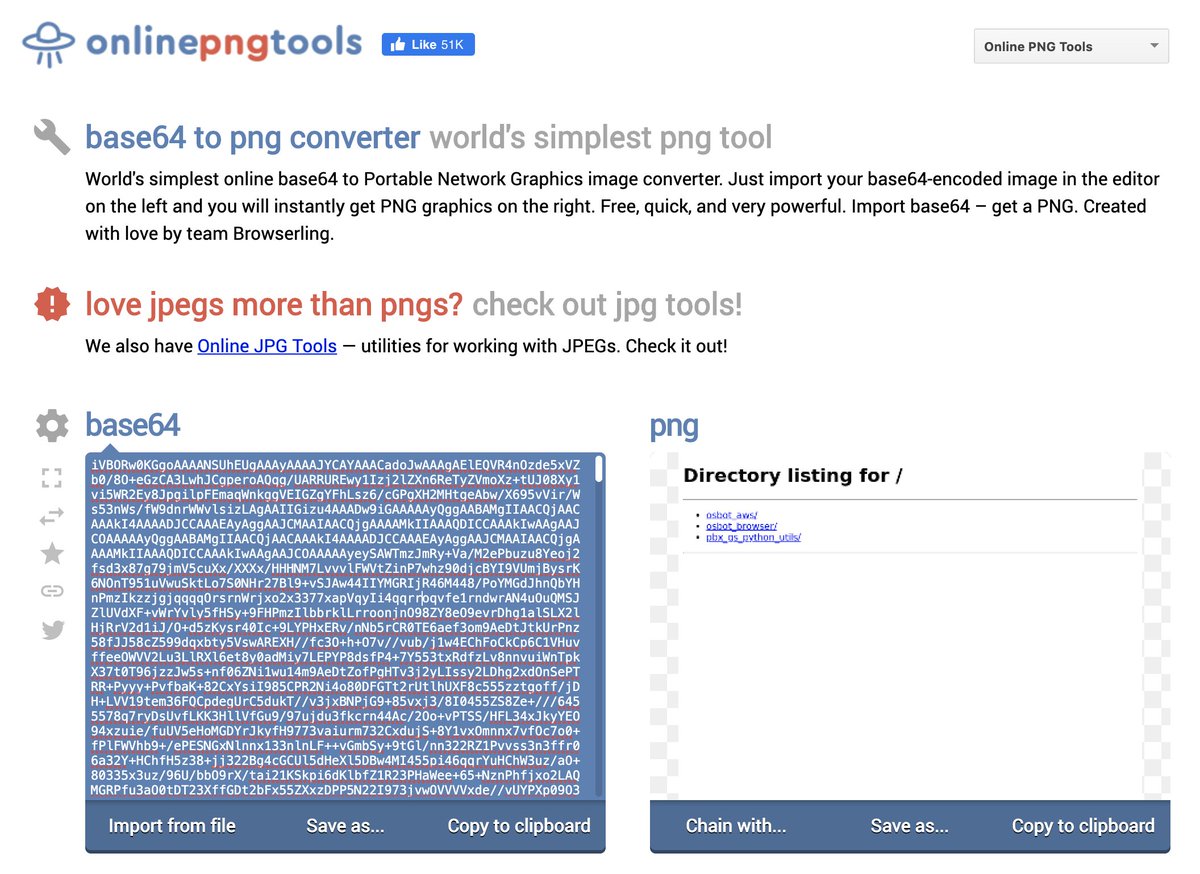
in this case, here is the 'wardley-maps/cup-of-tea.html' file which shows the typical example shown by @swardley when explaining wardley maps
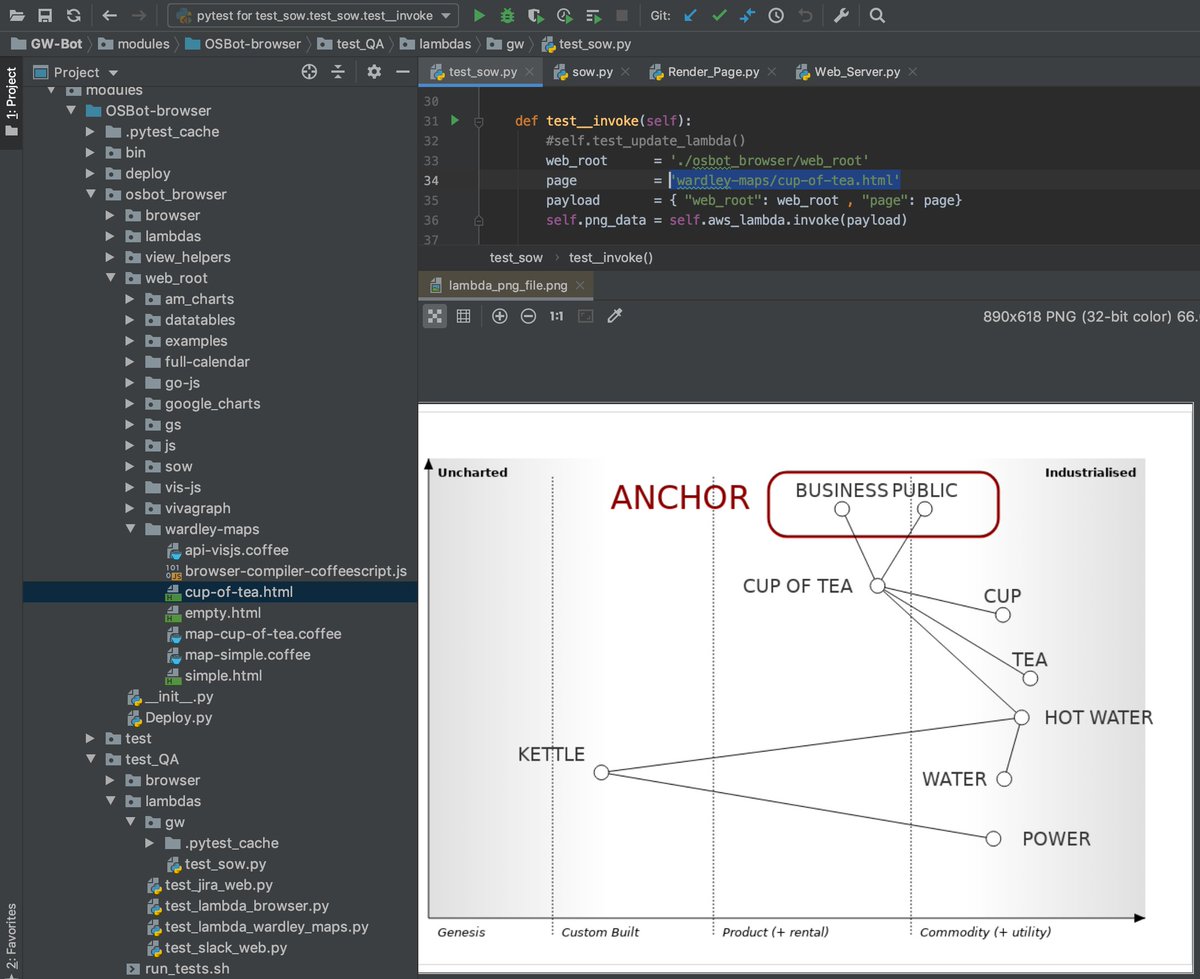
maps.add_component('TEA BAGS', 1.2, 5)
maps.add_connection('TEA', 'TEA BAGS')
@swardley @_tony_richards @HiredThought @madplatt @simonaclifford @Rachel0404
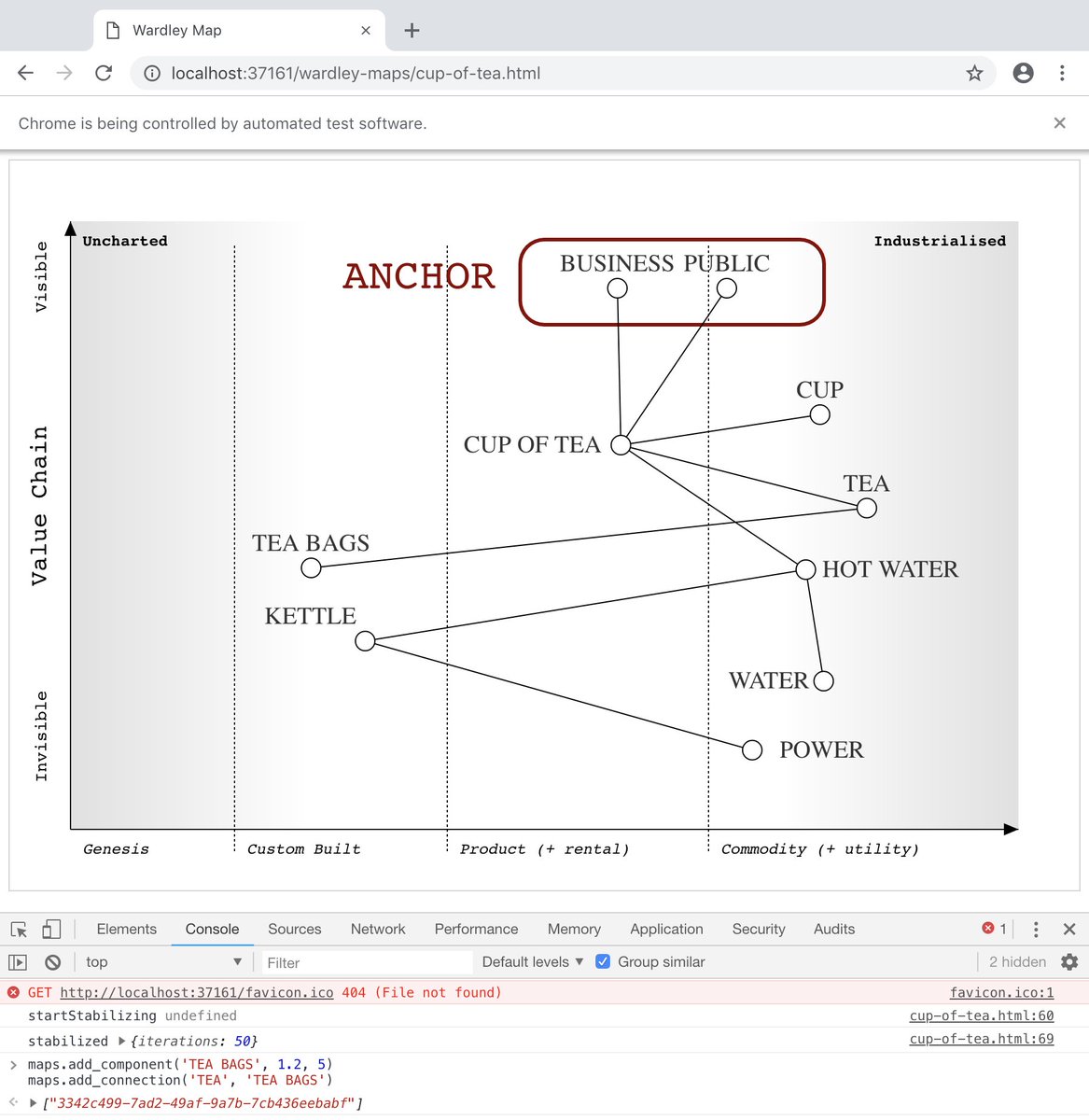
So here is how I have been developing lambda functions for the past year, in a super efficient workflow
All code is open sourced as part of the @OWASP Security Bot github.com/owasp-sbot/OSB…
Let me know if you have any questions or need clarification on some steps