I know that in some countries a degree in CS is expensive or unattainable, and that some companies do unnecessary algorithm interviews.
This thread is not about degrees or interviews, it's about CS itself.
It's vital to have some knowledge which is *indirectly* applied.
E.g. trees, graphs, data structures, graph search (breadth-first, depth-first), languages
- Array
- Object
- Set
- Map
- Simple directories in the file system
- JSON is a tree
- HTML is a tree
- DOM is a tree
Some data structures are "graphs" 🔗:
- JS Objects (can have circular refs)
- GraphQL ( graphql.org/learn/thinking… )
- Array has push/pop
- Object has get/set through [ ] brackets
- DOM has appendChild etc
- GraphQL has mutations
It's great if you can learn strategies for trees so you once you know how handle JSON, you can handle the DOM, both are trees.
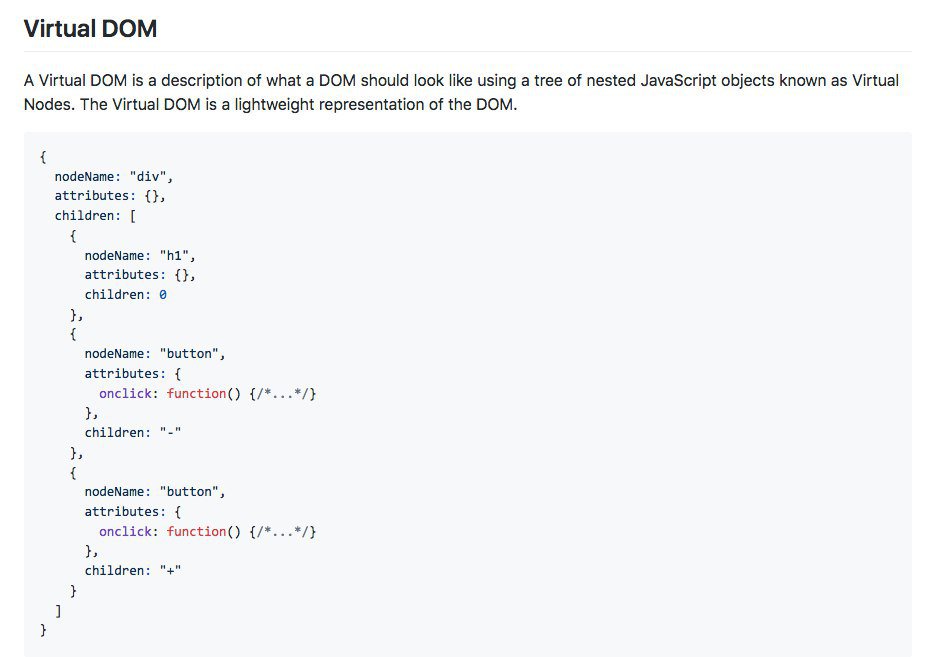
npm install does an advanced graph search, while creating a *tree* (package-lock.json). Usually if some processing takes a lot of time (npm i), creating a temporary data structure (package-lock) helps.
Computation can be light/heavy in two basic dimensions: time spent versus storage spent. There's a whole subfield dedicated to heaviness.
- Depth-first (prioritize going deep)
- Breadth-first (prioritize going evenly through all children)
Maybe you've found a bug once where one of these modes was the wrong choice for the use case.
github.com/ReactiveX/rxjs…
With some knowledge of formal languages, syntactical analysis, grammars, and compilers you could save hours of regex attempts by knowing that it's mathematically impossible to parse HTML with regex.
I don't want anyone to feel pressured to get a degree, I just want to encourage reading on Wikipedia basic CS concepts.
Have a nice week! 💾
Ever built an array B based on another array A?
const B = []
for (let x of A)
B.push(`${x} €`)
That's a "map" operation:
B = A.map(x => `${x} €`)
const B = []
for (let x of A)
if (x < 100)
B.push(x)
That's a "filter" operation:
B = A.filter(x => x < 100)
- SELECT == map
- WHERE == filter
Oh cool that's half way to recreating SQL
It's rooted in relational algebra.