Basically, i would sign up interested people by tagging them, and then step-by-step show them how to build the app. All in public.
Anyone interested?
To install Node: nodejs.org/en/download/pa…
Windows: Windows+R, “cmd.exe”
Linux: console (ctrl+alt+A)
macOS: Spotlight, “terminal”
This is a bit of an experiment. I don't know how well it will work. We'll all learn together ... in public. :-)
@JS_Jedi
@innthomas
@blackbeardcoder
@Fallenstedt
If you want to be added into the mix, let me know and I'll tag you in future tweets.
STEP 1
Navigate to a folder on your computer where you keep your experimental projects. Mine is ~/Code/Temp
A. "Like" each tweet when you've done the step.
B. If you need help, reply to the relevant tweet.
New peeps: @edelman215 👋
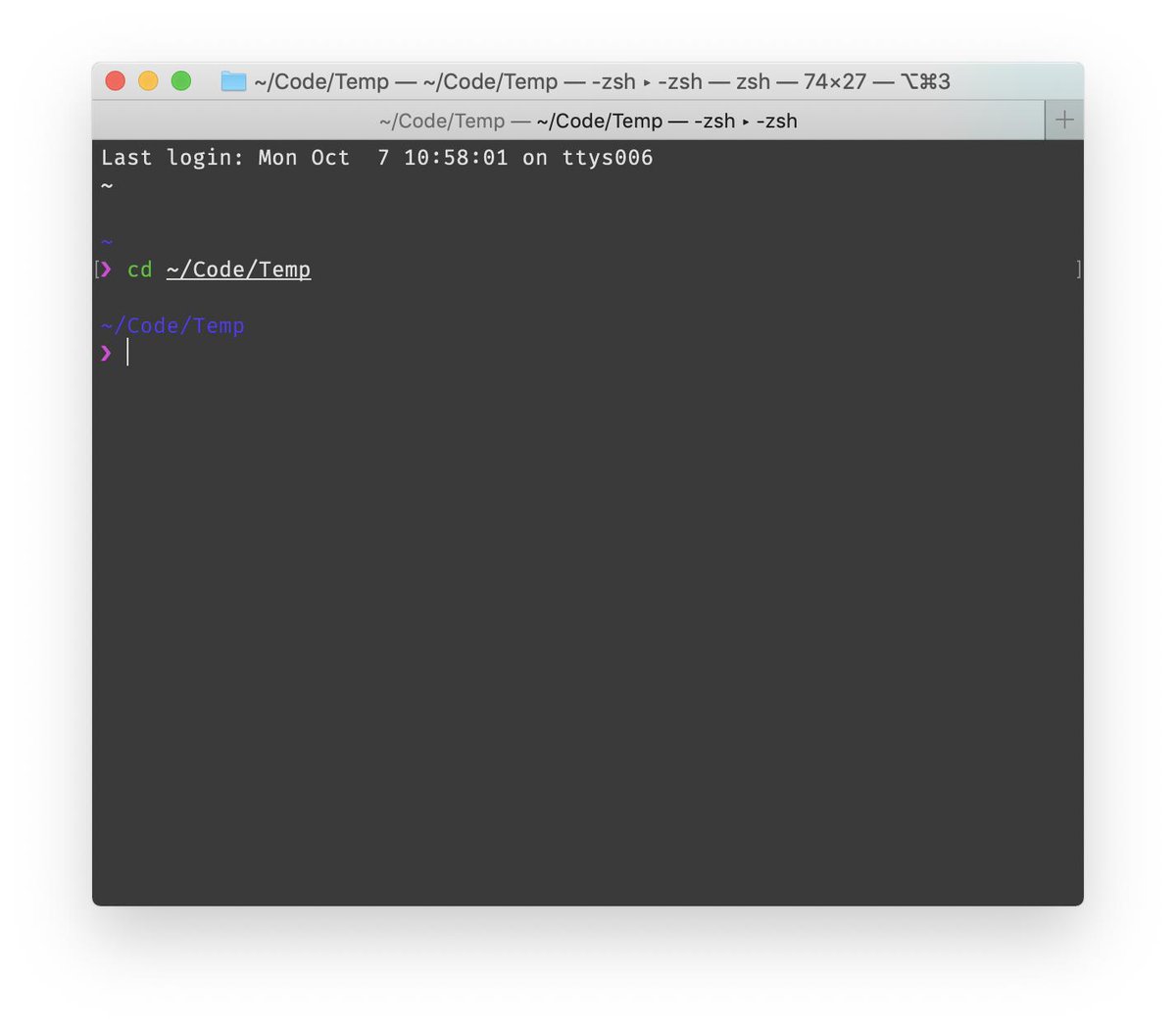
Let's use `npx` to run Gluegun's CLI, which will create a new CLI for us to modify.
npx lets you run CLIs without installing them globally. It's part of Node/NPM.
npx gluegun new codecounter
Choose TypeScript. Yes, we'll be using TypeScript for this. 😇
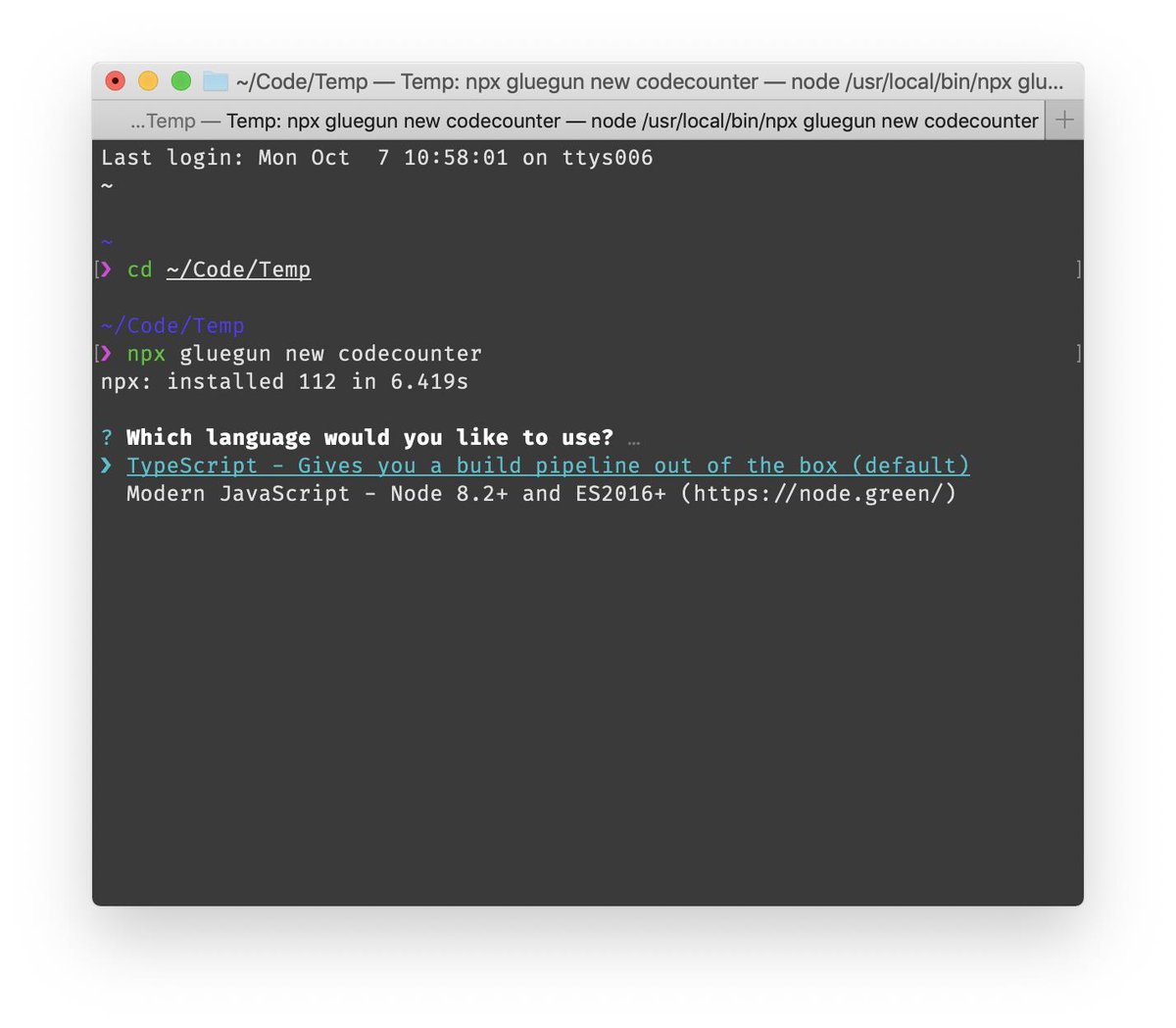
If all goes well, you should see something like this. CD into the folder and run `yarn test` and `yarn link`.
If you don't have yarn, run `npm test` and `npm link`. They should work the same.
Ignore the part about `yarn build`, we won't be doing that ... at least now.
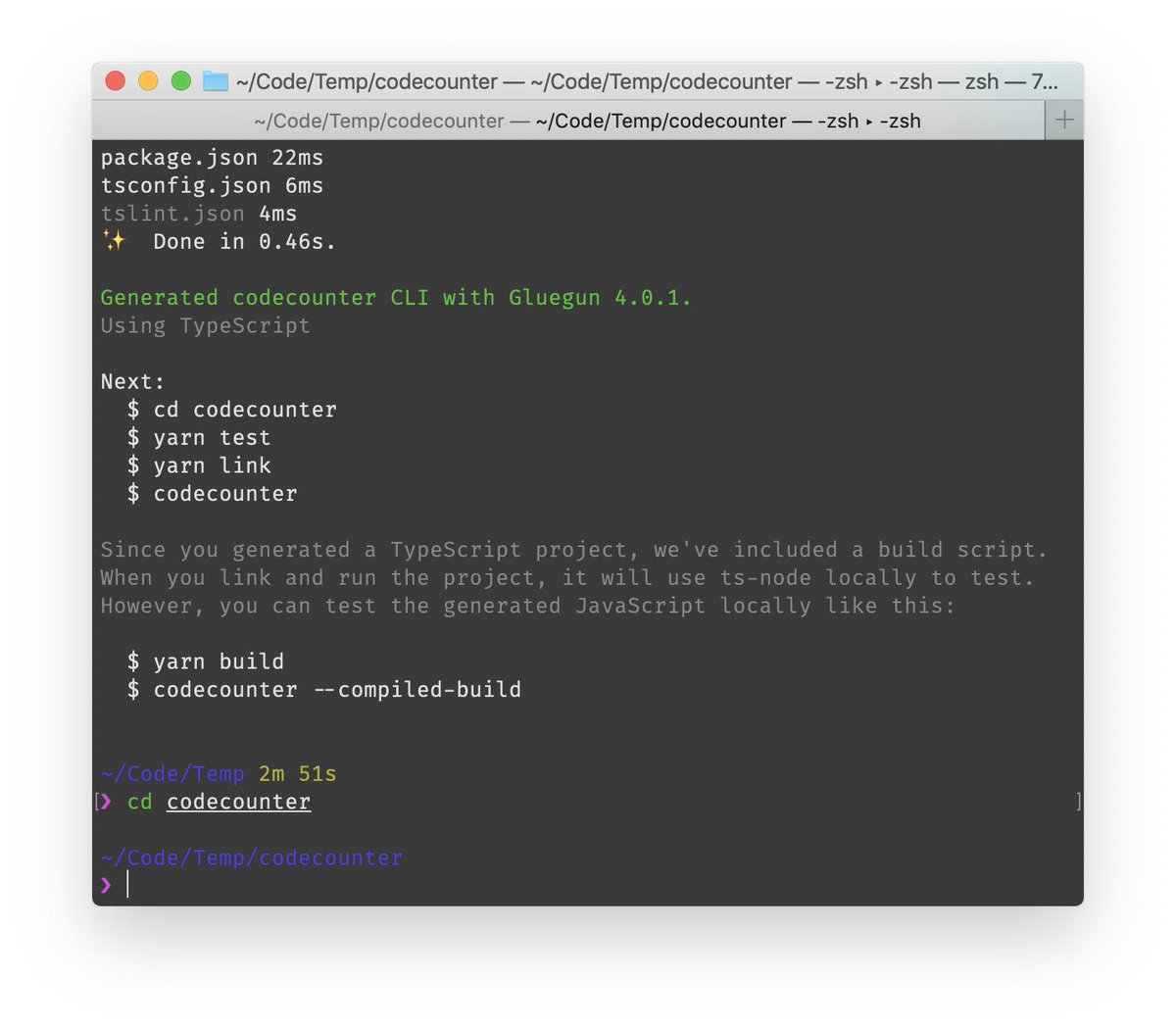
I ran into a test failure. This is a Gluegun bug that I'm aware of and in the process of fixing, but let's go ahead and fix it now in our CLI.
Open the app in your favorite editor. I use VS Code, so I go:
code .
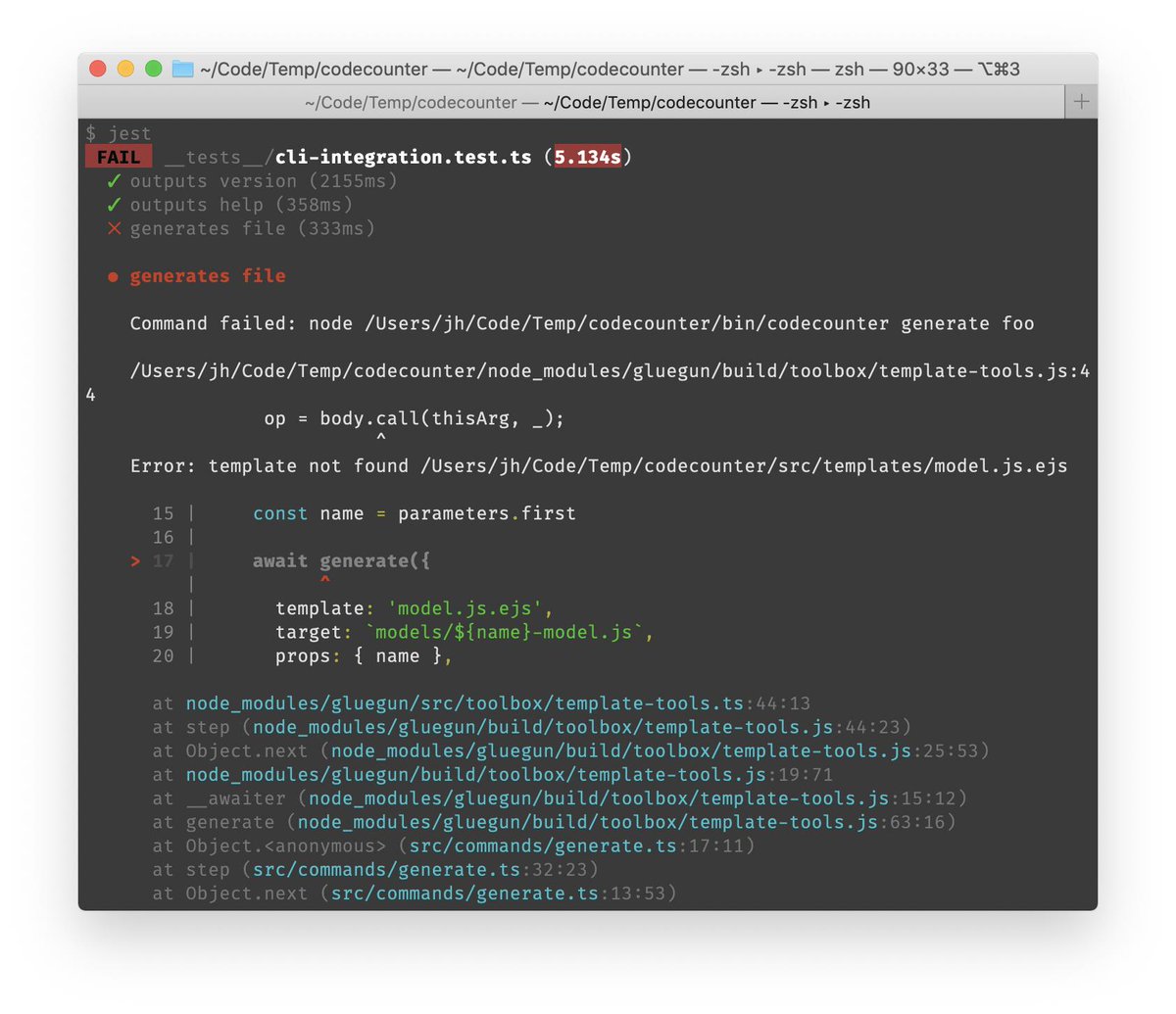
We don't really even need the command that is failing. So let's remove it from our tests.
Go to __tests__/cli-integration.test.js and remove this highlighted section. Re-run your tests and they should pass.
yarn test
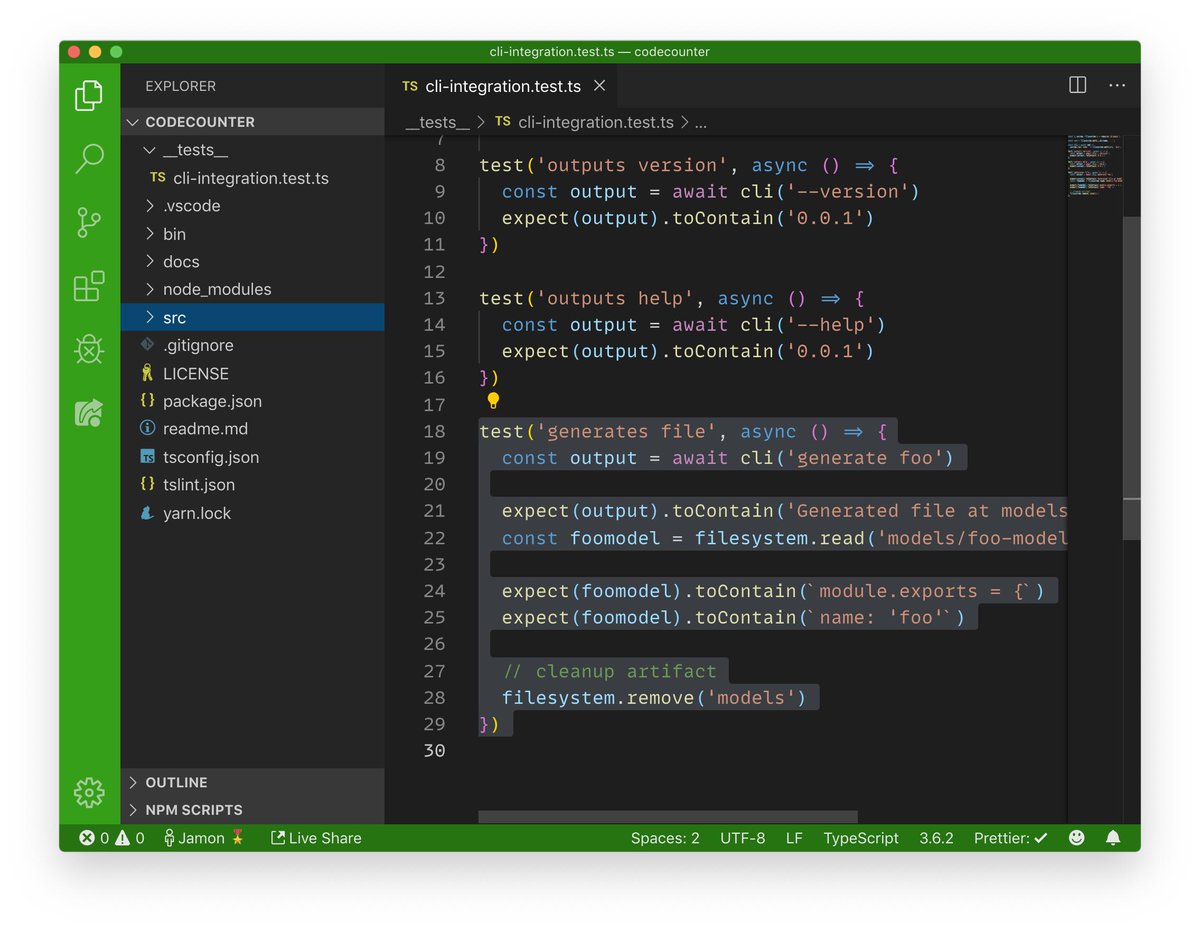
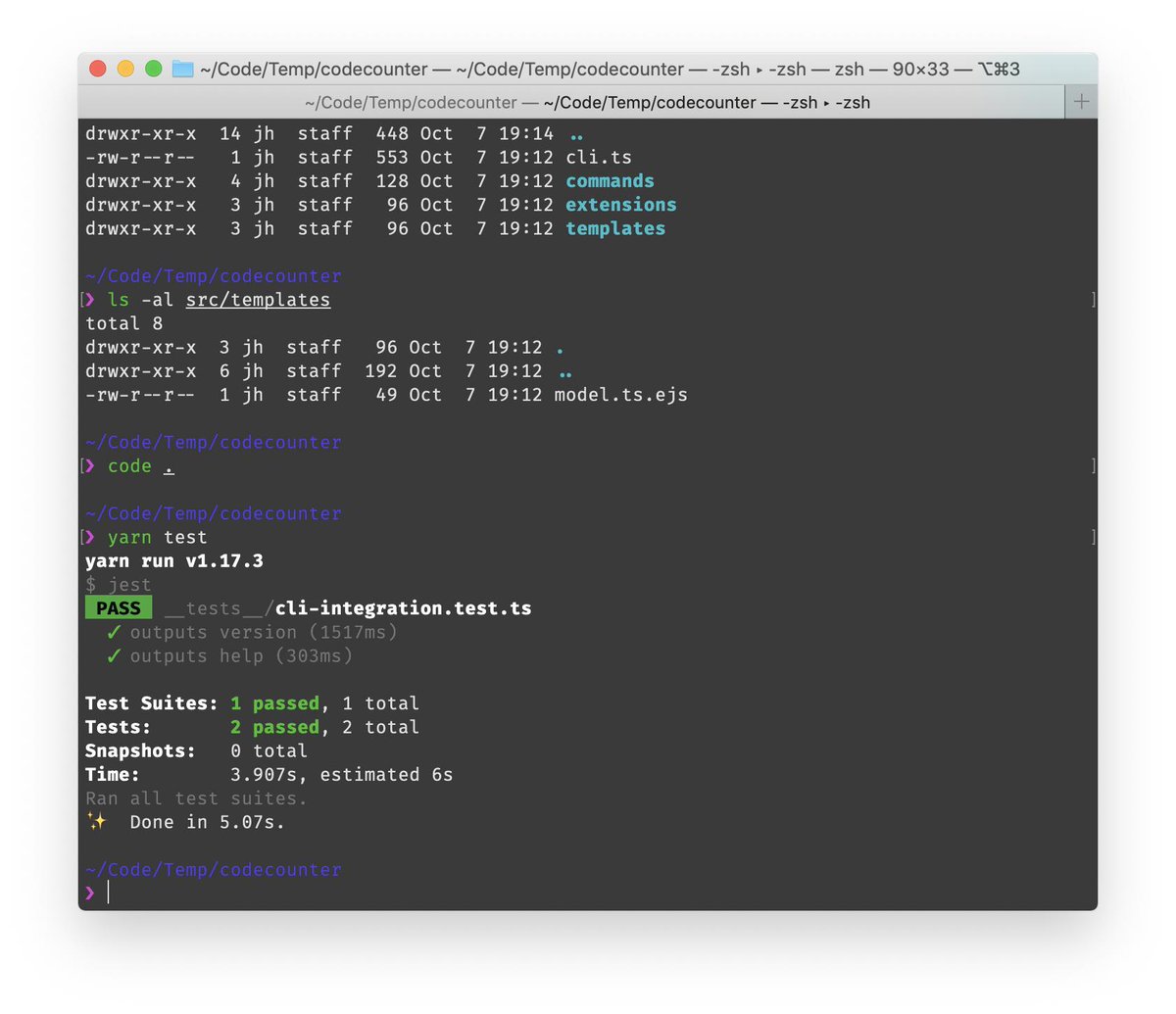
😂
Onward...
Gluegun ships with 3 different folders in the src directory: commands, extensions, and templates.
We won't be using the templates folder or the `generate.ts` command, so you can safely remove those.
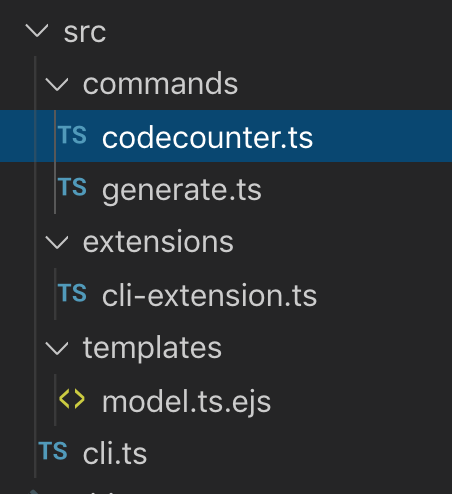
If you've run the `yarn link` / `npm link` step, you should be able to run your CLI like this:
codecounter
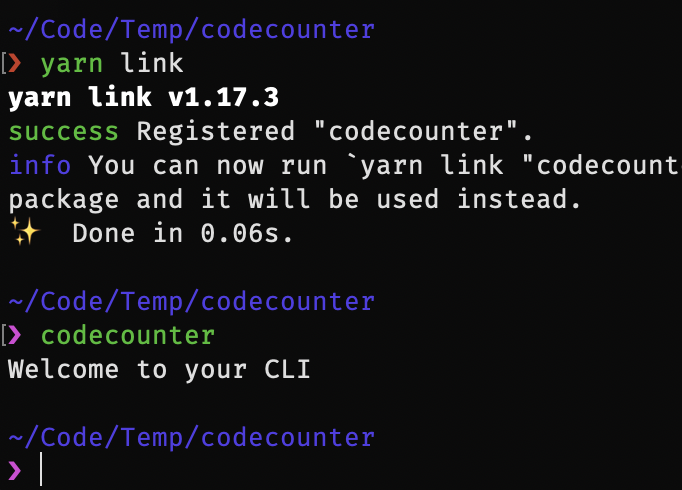
Let's modify that default command to see how it works.
print.info('CodeCounter Version ' + toolbox.meta.version())
Re-running your command should show the version.
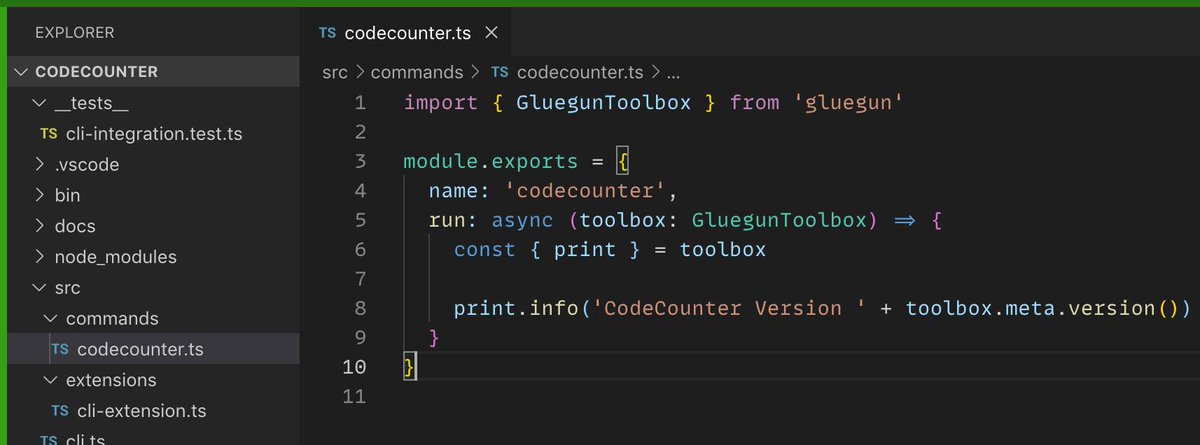
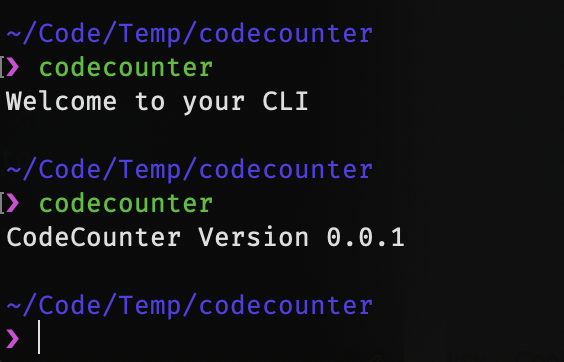
This is a good time to introduce the Gluegun "toolbox". You'll be using a lot of its tools as you build out your CLI, so check out the docs here:
github.com/infinitered/gl…
Let's make a new command called "codecounter count". This will take a folder name and then recursively walk up the tree to find all files with the languages we care about.
Just make a new file in ./src/commands called "count.ts" and add the following.
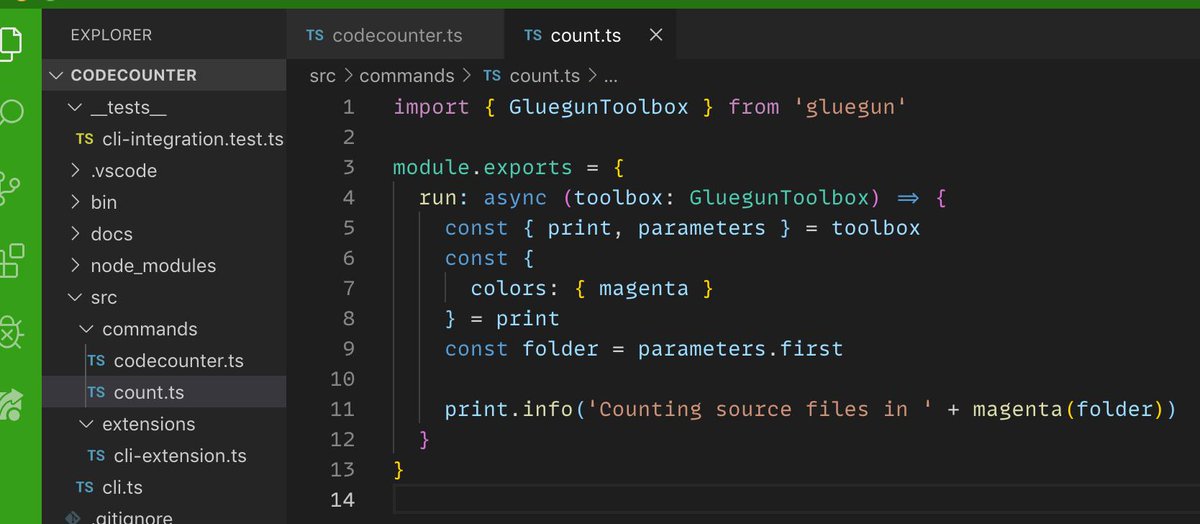
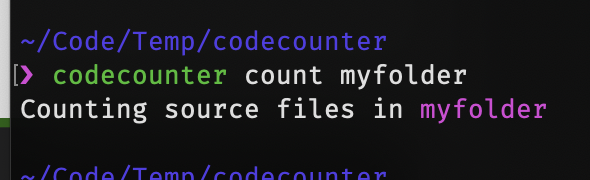
Now let's actually list out files in that folder.
We'll use the filesystem.find command:
github.com/infinitered/gl…
Modify the command to import the filesystem tools and use the command to find files. If you run it against our own ./src folder, you'll get the 3 TS files.
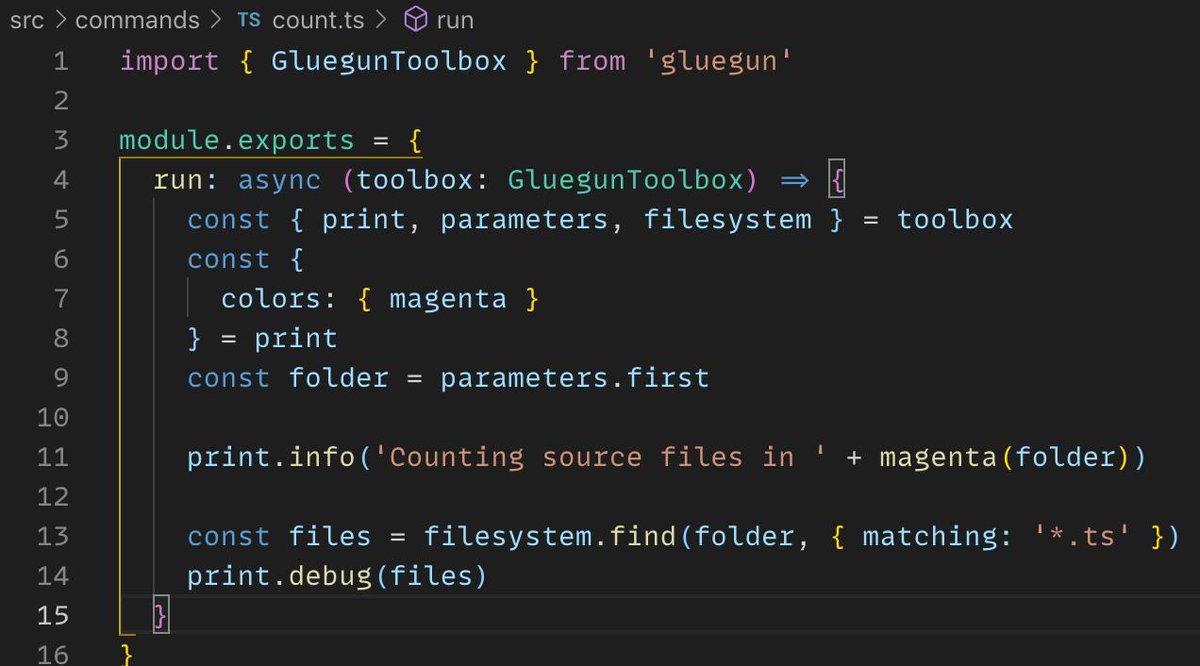
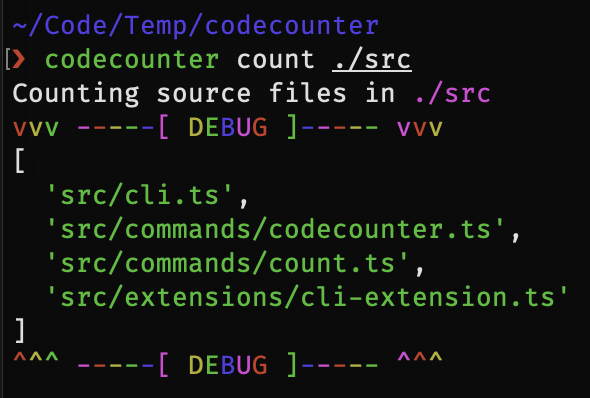
We don't just want typescript files, though. Make a few dummy HTML and CSS files in your src folder and then modify the `matching` option to be:
matching: '*(*.ts|*.html|*.css)'
I made it its own variable so it's easier to modify later.
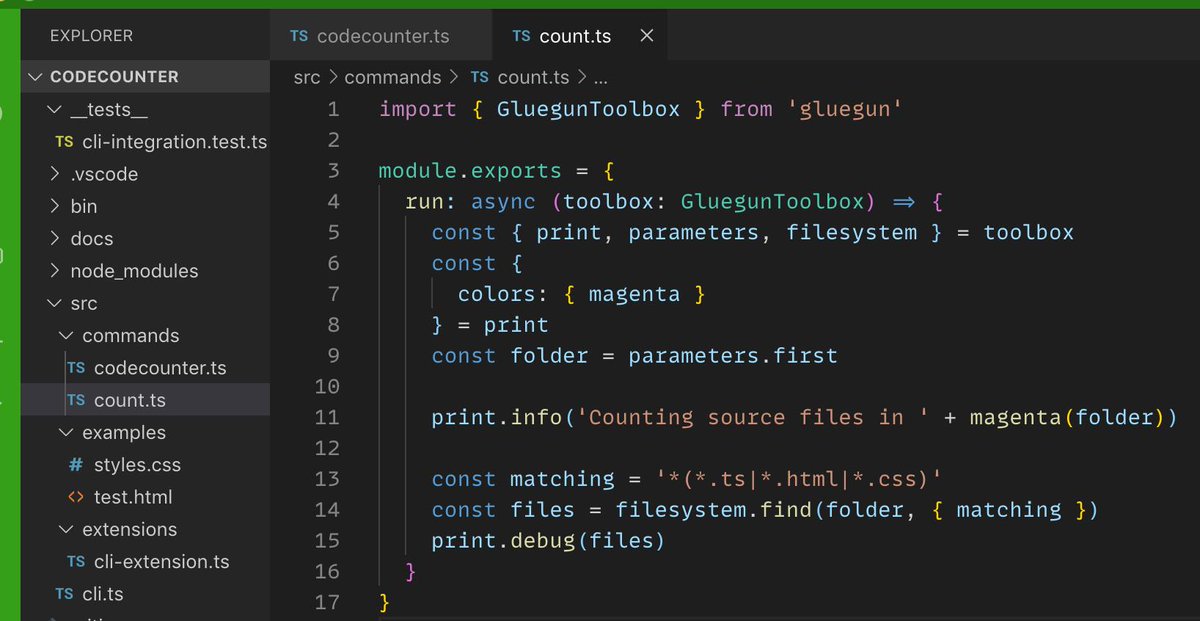
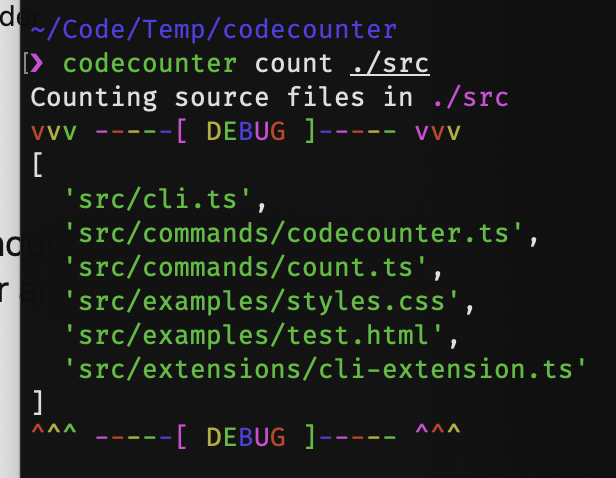
Now let's count all the lines of code in each file and get the result.
I was going to be unnecessarily clever here and use a reduce function, but -- nah, let's use a forEach.
Here I loop over the files, check the extension, and next we will need to increment each type.
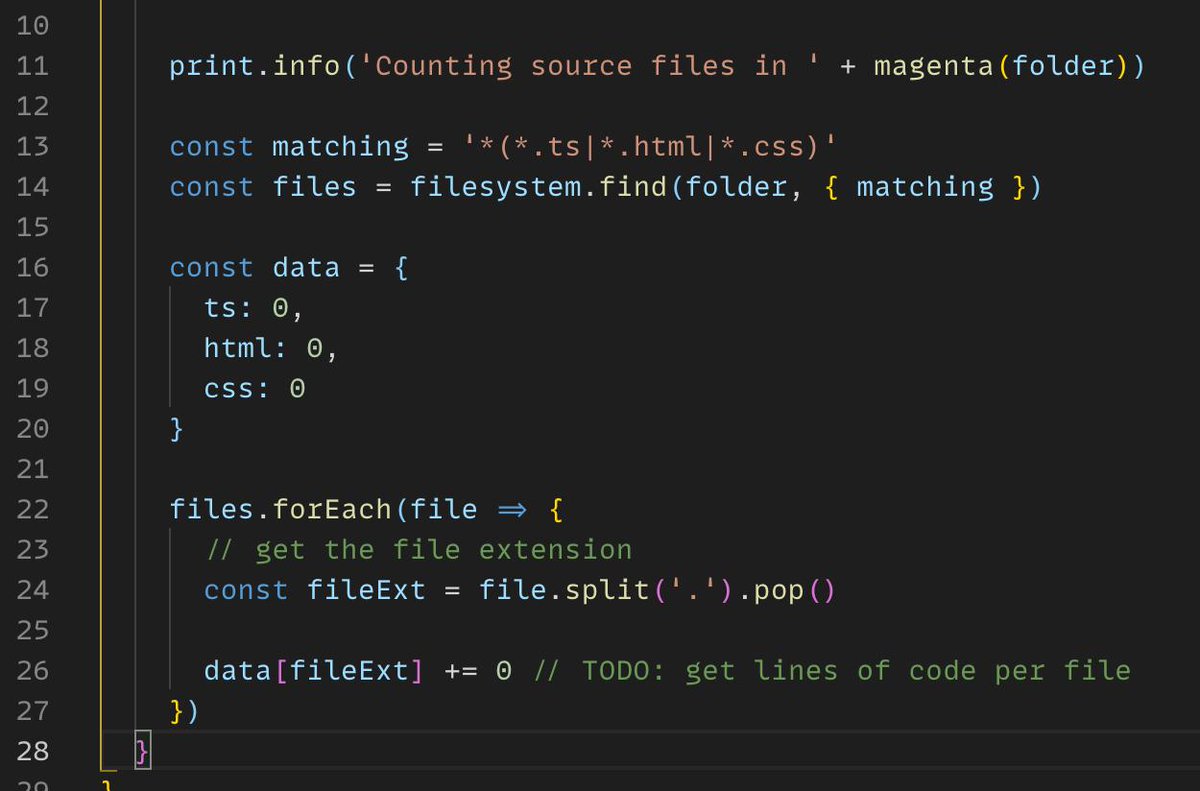
We use the `filesystem.read()` tool to read in the contents of each file, and then count the lines by splitting by line breaks and counting how many segments there are.
github.com/infinitered/gl…
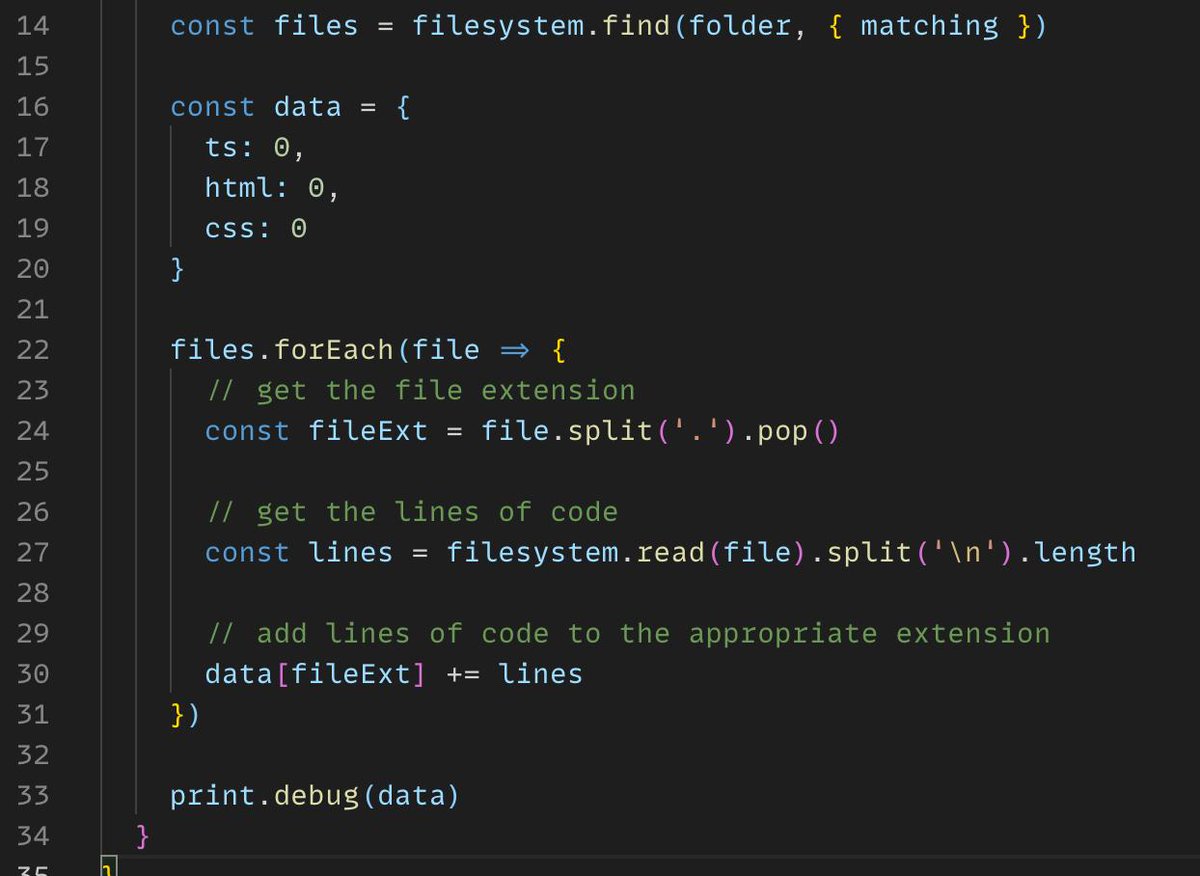
If you run the command, you should see something like this.
Cool! We have the data. But it needs to be formatted.
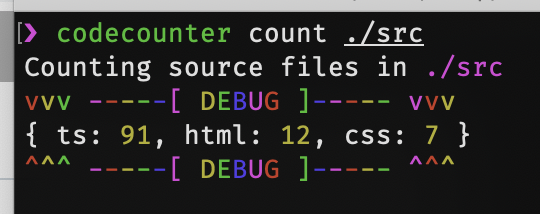
@codefriar asked about how we would use the reduce function here. Let's do it!
As you can see, it's not really much cleaner here. There are some cases where it feels cleaner and shorter but this isn't one of them.
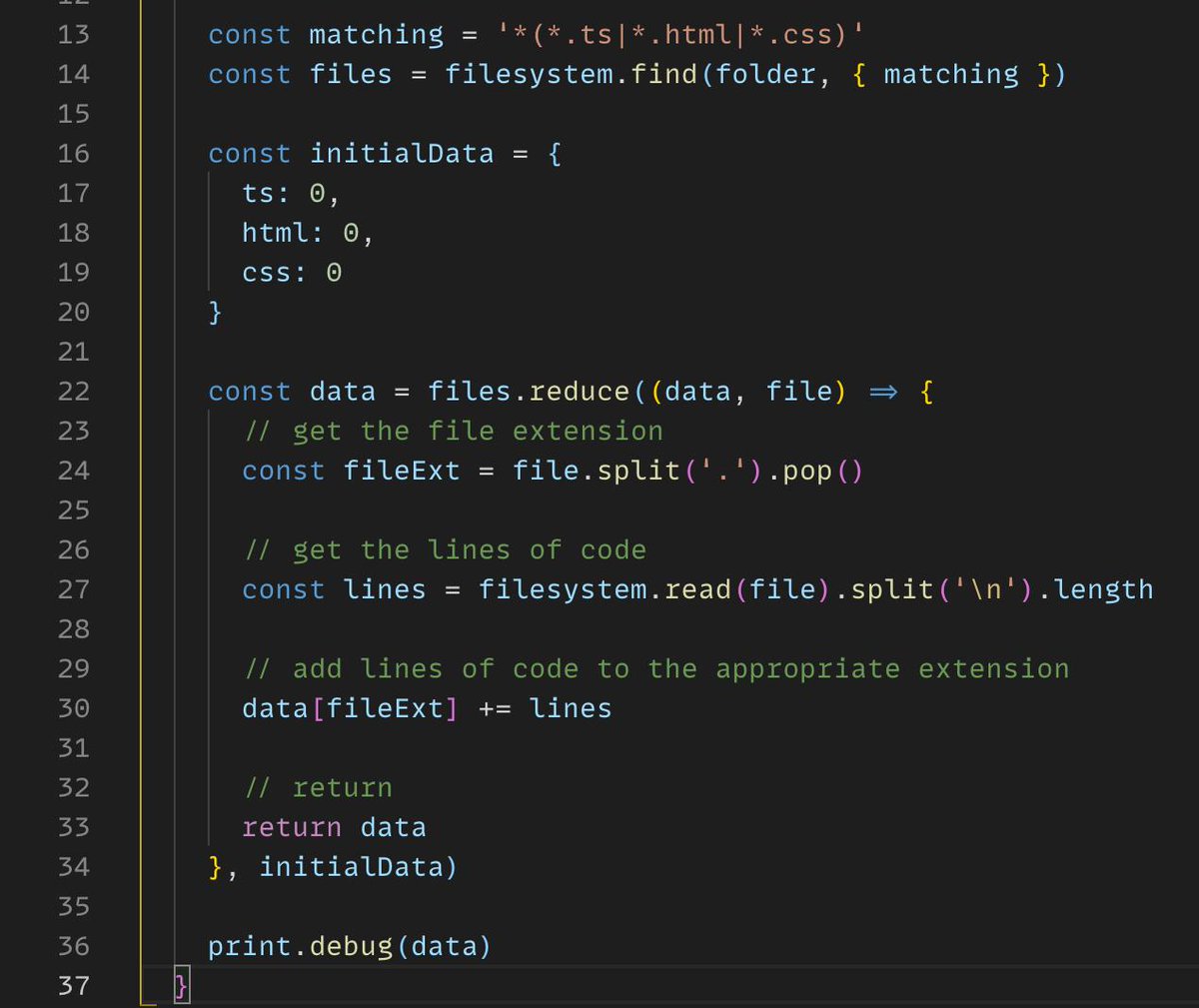
Let's format the data.
Gluegun comes with a table printer, print.table.
github.com/infinitered/gl…
We're going to format the table as "lean" and also use Object.entries as a quick way to separate the key/value to arrays of 2 (tuples).
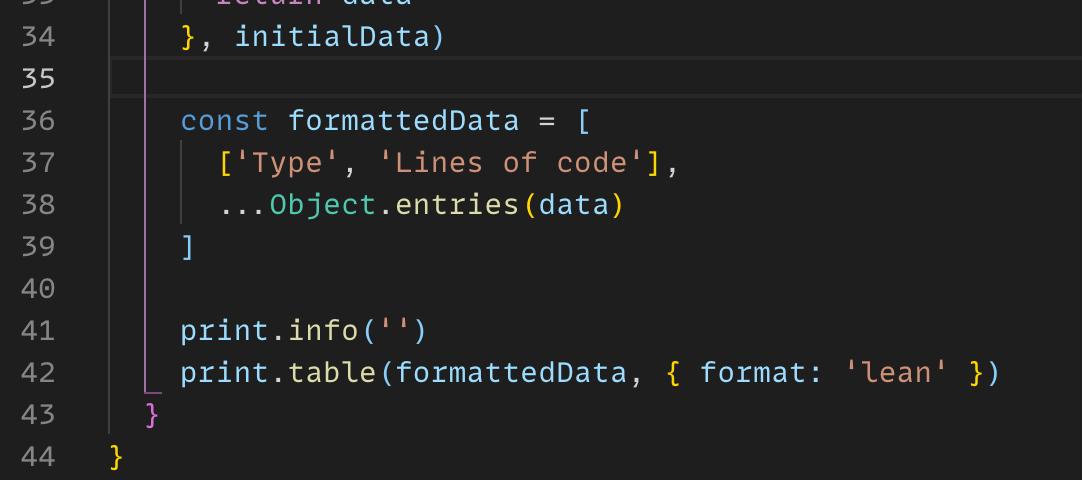
We're done!! Try it out on your node_modules:
Post your screenshot!
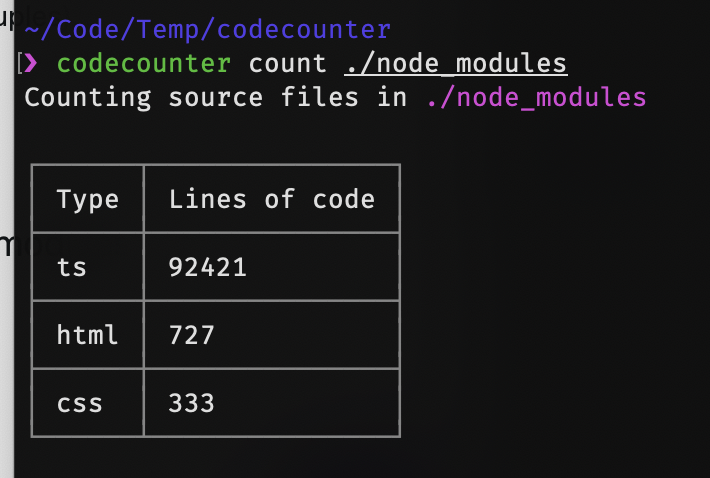
1. Try sorting your data from most to least
2. Try adding JS, Markdown, and other source file extensions
4. Translate the simple file extensions to their full names in the table
5. Allow passing in file extensions, like:
codecounter count ./src --types "js|css|swift|go"
Hint: check `toolbox.parameters.options`!
Other ideas?
Not a lot.
But it was there, keeping an eye on things regardless!